查找子串,补充下面的程序 #include<stdio.h> #include<string.h> int fun(char *s,char*t) { // } int main() { char str1[30],str2[10]; gets(str1); gets(str2); printf("%d",fun(str1,str2)); return 0; }
时间: 2024-01-12 21:02:43 浏览: 58
可以使用字符串库函数strstr来查找子串,具体实现如下:
```c
#include<stdio.h>
#include<string.h>
int fun(char *s, char *t) {
char *p = strstr(s, t); // 在s中查找t
if (p == NULL) { // 如果找不到,返回-1
return -1;
} else { // 如果找到,返回t在s中的起始位置
return p - s;
}
}
int main() {
char str1[30], str2[10];
gets(str1);
gets(str2);
printf("%d", fun(str1, str2));
return 0;
}
```
注意,gets()函数存在安全性问题,建议使用fgets()函数代替。
相关问题
#include <stdio.h> #include <string.h> void fun(char *s,int num) { } void main() { char s[10]; gets(s); fun(s,7); printf("%s", s); }
这是一道需要填空的题目,题目思路是将字符串 `s` 中的前 `num` 个字符反转。可以使用两个指针分别指向字符串的首位和末位,依次交换两个指针所指向的字符,直到两个指针相遇为止。具体实现可以这样写:
```c
#include <stdio.h>
#include <string.h>
void fun(char *s, int num) {
int i = 0, j = num - 1;
while (i < j) {
char temp = s[i];
s[i] = s[j];
s[j] = temp;
i++;
j--;
}
}
void main() {
char s[10];
gets(s);
fun(s, 7);
printf("%s", s);
}
```
其中,`i` 和 `j` 分别表示指向字符串的首位和末位的指针,`temp` 是一个临时变量,用于交换两个指针所指向的字符。在循环中,每次将指针向中间移动一位,并交换两个指针所指向的字符,直到两个指针相遇为止。最终输出交换后的字符串即可。
请使用while函数将fun函数的内容补充完整,编写出一个C语言程序,对字符串,除首、尾字符外,将其字符按ASCII编码数值降序排列的操作:#include<stdio.h> #include<ctype.h> #include<string.h> #include<conio.h> void wwjt(); void fun(char *a,int len) { int i,j; char t; } main(){ char s[100]; int l; printf("输入字符串:"); gets(s); l=strlen(s); fun(s,l); printf("\n%s\n",s); wwjt(); }
#include<stdio.h>
#include<ctype.h>
#include<string.h>
#include<conio.h>
void wwjt();
void fun(char *a, int len) {
int i, j;
char t;
i = 1;
j = len - 2; //除去首尾字符后的最后一个字符的下标
while (i < j) {
while (i < j && a[i] > a[j]) {
t = a[i];
a[i] = a[j];
a[j] = t;
i++;
j--;
}
i++;
j--;
}
}
int main() {
char s[100];
int l;
printf("输入字符串:");
gets(s);
l = strlen(s);
fun(s, l);
printf("\n%s\n", s);
wwjt();
return 0;
}
void wwjt() {
printf("我爱计算机网络课程设计!\n");
}
阅读全文
相关推荐
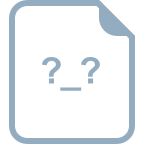












