人工智能归结原理实验代码python
时间: 2023-06-11 13:08:14 浏览: 196
以下是一个简单的人工智能归结原理实验代码,使用Python编写:
```
import re
def unify(x, y, theta):
if theta is None:
return None
elif x == y:
return theta
elif isinstance(x, str) and x[0].islower():
return unify_var(x, y, theta)
elif isinstance(y, str) and y[0].islower():
return unify_var(y, x, theta)
elif isinstance(x, list) and isinstance(y, list):
return unify(x[1:], y[1:], unify(x[0], y[0], theta))
else:
return None
def unify_var(var, x, theta):
if var in theta:
return unify(theta[var], x, theta)
elif x in theta:
return unify(var, theta[x], theta)
else:
theta[var] = x
return theta
def subst(theta, clause):
if theta is None:
return None
elif isinstance(clause, list):
return [subst(theta, c) for c in clause]
elif isinstance(clause, str) and clause[0].islower():
return subst_var(theta, clause)
else:
return clause
def subst_var(theta, var):
if var in theta:
return subst(theta, theta[var])
else:
return var
def resolve(c1, c2):
for l1 in c1:
for l2 in c2:
theta = unify(l1, negate(l2), {})
if theta is not None:
resolvent = [subst(theta, c1_l) for c1_l in c1 if c1_l != l1] + \
[subst(theta, c2_l) for c2_l in c2 if c2_l != l2]
yield resolvent
def negate(literal):
if literal[0] == "~":
return literal[1:]
else:
return "~" + literal
def is_variable(s):
return isinstance(s, str) and s[0].islower()
def is_constant(s):
return isinstance(s, str) and s[0].isupper()
def parse_input(s):
clauses = []
for line in s.splitlines():
clause = []
for literal in re.findall(r'\w+', line):
if literal[0].isupper():
literal_type = 'constant'
else:
literal_type = 'variable'
clause.append((literal, literal_type))
clauses.append(clause)
return clauses
def main():
input_str = '''man(x)
woman(x)
loves(x, y)
loves(y, x)'''
goal = [('loves', 'variable', 'z')]
clauses = parse_input(input_str)
for i, clause in enumerate(clauses):
print(f"Clause {i}: {clause}")
new = set([tuple(c) for c in clauses])
while True:
old = new
new = set()
for c1 in old:
for c2 in old:
if c1 != c2:
for resolvent in resolve(c1, c2):
resolvent = tuple(resolvent)
if resolvent not in new and resolvent not in old:
new.add(resolvent)
print(f"New clause: {resolvent}")
for goal_literal in goal:
if unify(goal_literal, resolvent, {}) is not None:
print("Goal achieved!")
return
if __name__ == '__main__':
main()
```
这个代码演示了一些基本的人工智能归结原理操作,包括:
- `unify` 函数:用于统一两个项,即将它们赋予相同的值;
- `subst` 函数:用于将一个子句中的变量替换为它们的统一值;
- `resolve` 函数:用于对两个子句进行归结,生成归结式;
- `negate` 函数:用于将一个文字取反;
- `parse_input` 函数:用于将输入字符串解析为子句列表;
- `main` 函数:用于执行归结过程,直到达到目标。
该代码不包含完整的人工智能归结原理算法实现,但可以作为一个基础框架,让你开始编写自己的代码。
阅读全文
相关推荐
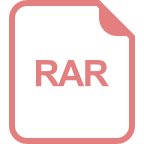
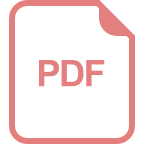
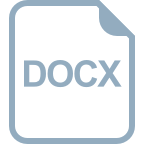
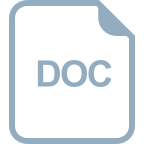
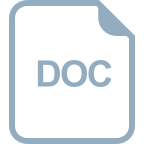
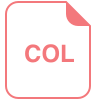
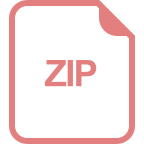
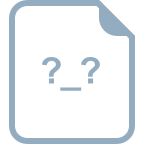
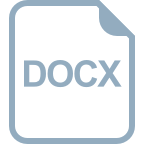
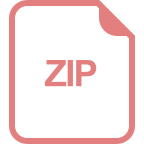
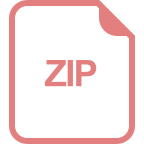