假定某班有40个同学参加了计算机等级考试,成绩(整数)从键盘输入,编写一个程序求该班考试最高分、最低分及平均分。
时间: 2023-05-31 14:20:59 浏览: 152
### 回答1:
好的,以下是用中文回复:
假设有40个同学参加了计算机等级考试,他们的成绩(整数)从键盘输入。为了求出该班考试的最高分、最低分和平均分,我们可以编写一个程序。
首先,我们需要定义一个数组来存储这40个同学的成绩。然后,我们可以使用循环语句来遍历这个数组,找到最高分和最低分,并计算出所有成绩的总和。最后,我们可以用总和除以40来得到平均分。
下面是一个示例程序:
#include <stdio.h>
int main() {
int scores[40];
int i, max, min, sum = ;
float avg;
// 从键盘输入40个同学的成绩
for (i = ; i < 40; i++) {
printf("请输入第%d个同学的成绩:", i+1);
scanf("%d", &scores[i]);
}
// 找到最高分和最低分
max = scores[];
min = scores[];
for (i = 1; i < 40; i++) {
if (scores[i] > max) {
max = scores[i];
}
if (scores[i] < min) {
min = scores[i];
}
}
// 计算总分和平均分
for (i = ; i < 40; i++) {
sum += scores[i];
}
avg = (float)sum / 40;
// 输出结果
printf("最高分:%d\n", max);
printf("最低分:%d\n", min);
printf("平均分:%.2f\n", avg);
return ;
}
希望这个程序能够帮助你解决问题!
### 回答2:
为了求出某班40个同学参加计算机等级考试的最高分、最低分和平均分,我们需要按照以下步骤进行编写程序。
1. 定义变量
在程序开始时,需要定义保存学生成绩的变量。可以使用一个整型数组来保存40个同学的成绩数据,同时设置三个变量用于保存最高分、最低分和总分数,一个变量用于计算学生总数。
int scores[40], highest_score, lowest_score, total_score = 0, student_count = 0;
2. 输入并存储学生成绩
使用for循环来输入并存储学生成绩,并且在每次输入时累加总分数和学生数目。如果该成绩高于最高分,则更新最高分;如果该成绩低于最低分,则更新最低分。循环结束时,累加所有学生成绩,用于计算平均分。
for(int i=0; i<40; i++){
cout << "请输入第" << (i+1) << "个学生的成绩:" << endl;
cin >> scores[i];
total_score += scores[i];
student_count++;
if(scores[i] > highest_score){
highest_score = scores[i];
}
if(scores[i] < lowest_score){
lowest_score = scores[i];
}
}
3. 计算平均分并输出结果
用累加的总分除以学生数目得到平均分。最后输出最高分、最低分和平均分的结果。
float average_score = total_score / student_count;
cout << "最高分:" << highest_score << endl;
cout << "最低分:" << lowest_score << endl;
cout << "平均分:" << average_score << endl;
完整的程序如下:
#include <iostream>
using namespace std;
int main(){
int scores[40], highest_score = 0, lowest_score = 100, total_score = 0, student_count = 0;
for(int i=0; i<40; i++){
cout << "请输入第" << (i+1) << "个学生的成绩:" << endl;
cin >> scores[i];
total_score += scores[i];
student_count++;
if(scores[i] > highest_score){
highest_score = scores[i];
}
if(scores[i] < lowest_score){
lowest_score = scores[i];
}
}
float average_score = total_score / student_count;
cout << "最高分:" << highest_score << endl;
cout << "最低分:" << lowest_score << endl;
cout << "平均分:" << average_score << endl;
return 0;
}
### 回答3:
首先,我们可以声明一个长度为40的数组,用于存储所有40个同学的考试成绩。然后,可以通过循环,从键盘输入这40个同学的成绩,并将其依次存储到数组中。
接着,可以分别通过遍历数组的方式,求出该班考试的最高分、最低分及平均分。具体方法如下:
1. 求最高分:可以设置一个变量max_score,先将其赋值为数组中第一个元素,然后依次遍历数组中的每个元素,若某个元素的值大于max_score,则将max_score的值更新为当前元素的值,最终得到的max_score即为该班考试的最高分。
2. 求最低分:同理,可以设置一个变量min_score,先将其赋值为数组中第一个元素,然后依次遍历数组中的每个元素,若某个元素的值小于min_score,则将min_score的值更新为当前元素的值,最终得到的min_score即为该班考试的最低分。
3. 求平均分:可以设置一个变量sum_score,初始化为0,然后依次遍历数组中的每个元素,将每个元素的值累加到sum_score中。最终,将sum_score除以40就可以得到该班考试的平均分。
最后,可以输出求得的最高分、最低分和平均分即可。
相关推荐
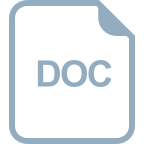
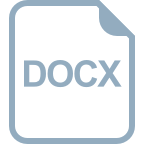














