用WGAN进行非平衡数据集的平衡处理,怎么用Python实现?
时间: 2023-08-17 15:16:10 浏览: 197
好的,以下是使用WGAN进行非平衡数据集的平衡处理的Python实现:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, BatchNormalization, LeakyReLU, Flatten, Reshape
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from sklearn.utils import shuffle
# 定义生成器
def make_generator(input_size):
input_layer = Input(shape=(input_size,))
x = Dense(128)(input_layer)
x = LeakyReLU(alpha=0.2)(x)
x = BatchNormalization()(x)
x = Dense(256)(x)
x = LeakyReLU(alpha=0.2)(x)
x = BatchNormalization()(x)
x = Dense(512)(x)
x = LeakyReLU(alpha=0.2)(x)
x = BatchNormalization()(x)
x = Dense(1024)(x)
x = LeakyReLU(alpha=0.2)(x)
x = BatchNormalization()(x)
output_layer = Dense(1, activation='sigmoid')(x)
return Model(input_layer, output_layer)
# 定义判别器
def make_discriminator():
input_layer = Input(shape=(1,))
x = Reshape((1,1))(input_layer)
x = Conv1D(64, 3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.2)(x)
x = Conv1D(128, 3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.2)(x)
x = Conv1D(256, 3, strides=2, padding='same')(x)
x = LeakyReLU(alpha=0.2)(x)
x = Flatten()(x)
output_layer = Dense(1)(x)
return Model(input_layer, output_layer)
# 定义Wasserstein Loss函数
def wasserstein_loss(y_true, y_pred):
return tf.reduce_mean(y_true * y_pred)
# 加载非平衡数据集
x_positive = np.load('positive_samples.npy')
x_negative = np.load('negative_samples.npy')
# 将数据集标准化
x_positive = (x_positive - x_positive.mean()) / x_positive.std()
x_negative = (x_negative - x_negative.mean()) / x_negative.std()
# 定义超参数
latent_dim = 100
batch_size = 128
epochs = 1000
n_critic = 5
clip_value = 0.01
# 创建生成器和判别器
generator = make_generator(latent_dim)
discriminator = make_discriminator()
# 编译判别器
discriminator.compile(optimizer=Adam(lr=0.0002, beta_1=0.5), loss=wasserstein_loss)
# 冻结判别器的权重
discriminator.trainable = False
# 定义GAN模型
input_layer = Input(shape=(latent_dim,))
output_layer = discriminator(generator(input_layer))
gan = Model(input_layer, output_layer)
# 编译GAN模型
gan.compile(optimizer=Adam(lr=0.0002, beta_1=0.5), loss=wasserstein_loss)
# 训练GAN模型
for epoch in range(epochs):
# 训练判别器
for _ in range(n_critic):
# 随机选择正样本和生成的负样本
idx = np.random.randint(0, len(x_positive), batch_size)
x_positive_batch = x_positive[idx]
x_negative_batch = generator.predict(np.random.normal(0, 1, (batch_size, latent_dim)))
# 训练判别器
discriminator_loss = discriminator.train_on_batch(np.concatenate([x_positive_batch, x_negative_batch]), np.concatenate([np.ones((batch_size, 1)), -np.ones((batch_size, 1))]))
# 截断判别器的权重
for layer in discriminator.layers:
weights = layer.get_weights()
weights = [np.clip(w, -clip_value, clip_value) for w in weights]
layer.set_weights(weights)
# 训练生成器
generator_loss = gan.train_on_batch(np.random.normal(0, 1, (batch_size, latent_dim)), np.ones((batch_size, 1)))
# 输出损失和进度
print("Epoch:", epoch, "Discriminator Loss:", discriminator_loss, "Generator Loss:", generator_loss)
```
这份代码使用了Wasserstein Loss作为损失函数,并使用WGAN进行非平衡数据集的平衡处理。在训练过程中,会先训练判别器,再训练生成器。判别器的权重会被截断,以避免梯度消失的问题。最终的输出是经过生成器生成的样本。
阅读全文
相关推荐
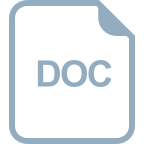
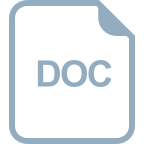
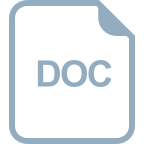

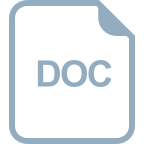
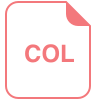
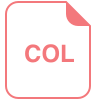
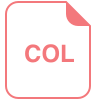
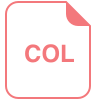
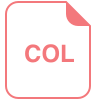
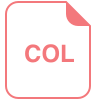
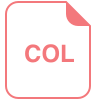
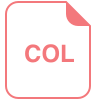
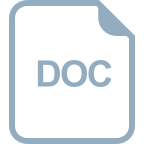
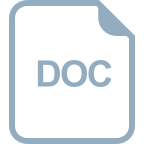
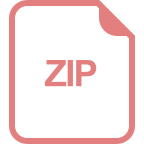
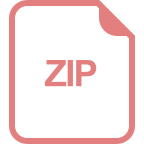
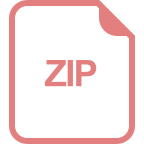
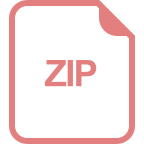