用java写一个商品库存管理系统的代码
时间: 2024-06-12 08:07:48 浏览: 15
抱歉,作为AI语言模型,我无法提供完整的代码。以下是一个简单的商品库存管理系统的Java类的示例代码,可供参考:
```
import java.util.Scanner;
public class InventoryManagementSystem {
private String[] products;
private int[] quantities;
public InventoryManagementSystem(int size) {
products = new String[size];
quantities = new int[size];
}
public void addProduct(String productName, int quantity) {
int index = findProduct(productName);
if (index == -1) {
index = findEmptyIndex();
if (index != -1) {
products[index] = productName;
quantities[index] = quantity;
System.out.println("Product added successfully.");
}
else {
System.out.println("Inventory is full.");
}
}
else {
quantities[index] += quantity;
System.out.println("Quantity updated successfully.");
}
}
public void sellProduct(String productName, int quantity) {
int index = findProduct(productName);
if (index != -1) {
if (quantities[index] >= quantity) {
quantities[index] -= quantity;
System.out.println("Sale recorded successfully.");
}
else {
System.out.println("Insufficient quantity in stock.");
}
}
else {
System.out.println("Product not found.");
}
}
public void displayInventory() {
System.out.println("Product\tQuantity");
for (int i = 0; i < products.length; i++) {
if (products[i] != null) {
System.out.println(products[i] + "\t" + quantities[i]);
}
}
}
private int findProduct(String productName) {
for (int i = 0; i < products.length; i++) {
if (products[i] != null && products[i].equalsIgnoreCase(productName)) {
return i;
}
}
return -1;
}
private int findEmptyIndex() {
for (int i = 0; i < products.length; i++) {
if (products[i] == null) {
return i;
}
}
return -1;
}
public static void main(String[] args) {
InventoryManagementSystem ims = new InventoryManagementSystem(10);
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter 1 to add a product, 2 to sell a product, 3 to display inventory, or 0 to exit:");
int choice = scanner.nextInt();
switch (choice) {
case 0:
System.exit(0);
case 1:
System.out.println("Enter the name of the product:");
String productName = scanner.next();
System.out.println("Enter the quantity of the product:");
int quantity = scanner.nextInt();
ims.addProduct(productName, quantity);
break;
case 2:
System.out.println("Enter the name of the product:");
productName = scanner.next();
System.out.println("Enter the quantity of the product:");
quantity = scanner.nextInt();
ims.sellProduct(productName, quantity);
break;
case 3:
ims.displayInventory();
break;
default:
System.out.println("Invalid input.");
}
}
}
}
```
相关推荐
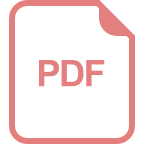
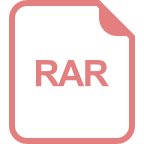














