将opc server的数据写入sqlserver
时间: 2023-11-30 22:02:13 浏览: 37
要将 OPC Server 的数据写入 SQL Server,您可以使用以下步骤:
1. 创建一个 OPC Server 的连接,以便从 OPC Server 读取数据。您可以使用 OPC DA 或 OPC UA 标准来与 OPC Server 进行通信。
2. 将读取的数据转换为 SQL Server 中的表格格式。
3. 使用 SQL Server 提供的 API(如 .NET Framework 提供的 SqlConnection 和 SqlCommand)来连接到 SQL Server 数据库并将数据写入相应的表格中。
下面是一个示例代码,展示如何使用 OPC DA 标准和 .NET Framework 的 SQL Server API 将 OPC Server 的数据写入 SQL Server 数据库中:
```csharp
using System;
using System.Data.SqlClient;
using Opc;
using Opc.Da;
class Program
{
static void Main(string[] args)
{
// Connect to OPC Server
var serverUrl = "opcda://localhost/Kepware.KEPServerEX.V6";
var opcServer = new Opc.Da.Server(new OpcCom.Factory(), new Opc.URL(serverUrl));
opcServer.Connect();
// Read data from OPC Server
var items = new Item[] { new Item { ItemName = "Random.Int1" }, new Item { ItemName = "Random.Real4" } };
var values = opcServer.Read(items);
// Convert data to table format
var dataTable = new System.Data.DataTable();
dataTable.Columns.Add("ItemName", typeof(string));
dataTable.Columns.Add("Value", typeof(object));
for (int i = 0; i < values.Length; i++)
{
dataTable.Rows.Add(items[i].ItemName, values[i].Value);
}
// Write data to SQL Server
var connectionString = "Data Source=MyServer;Initial Catalog=MyDatabase;Integrated Security=True";
using (var connection = new SqlConnection(connectionString))
{
connection.Open();
using (var command = connection.CreateCommand())
{
command.CommandText = "INSERT INTO MyTable (ItemName, Value) VALUES (@ItemName, @Value)";
command.Parameters.AddWithValue("@ItemName", "");
command.Parameters.AddWithValue("@Value", DBNull.Value);
foreach (var row in dataTable.Rows)
{
command.Parameters["@ItemName"].Value = row["ItemName"];
command.Parameters["@Value"].Value = row["Value"];
command.ExecuteNonQuery();
}
}
}
// Disconnect from OPC Server
opcServer.Disconnect();
}
}
```
请注意,此示例仅针对演示目的。在实际应用中,您需要根据您的数据源和目标数据库的具体情况进行相应的修改。
相关推荐
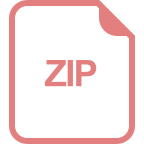














