springboot NtripServer
时间: 2023-10-30 15:07:11 浏览: 71
Spring Boot是一个流行的Java框架,可以帮助开发者快速构建基于Spring的应用程序。NtripServer是一种网络传输协议,常用于实现GPS数据传输。如果你想在Spring Boot中实现NtripServer,可以考虑使用Netty框架来实现网络传输部分,同时使用Spring Boot提供的注解来实现HTTP请求处理和路由。具体实现步骤如下:
1. 引入Netty和Spring Boot相关依赖
在`pom.xml`文件中引入以下依赖:
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.59.Final</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 实现NtripServer
使用Netty框架实现NtripServer的核心代码如下:
```java
public class NtripServer {
private final EventLoopGroup bossGroup;
private final EventLoopGroup workerGroup;
public NtripServer() {
bossGroup = new NioEventLoopGroup();
workerGroup = new NioEventLoopGroup();
}
public void start() throws InterruptedException {
try {
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpRequestDecoder());
pipeline.addLast(new HttpResponseEncoder());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new NtripServerHandler());
}
});
ChannelFuture future = bootstrap.bind(2101).sync();
future.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws InterruptedException {
new NtripServer().start();
}
}
```
这里使用了Netty提供的`ServerBootstrap`类来创建NtripServer,其中`bossGroup`和`workerGroup`分别是主线程池和工作线程池。在`childHandler`方法中,我们添加了Netty提供的HTTP相关的解码器和编码器,并将请求转发给`NtripServerHandler`进行处理。
3. 实现NtripServerHandler
`NtripServerHandler`可以继承Netty提供的`SimpleChannelInboundHandler`类,重写`channelRead0`方法来处理请求。在这个方法中,我们可以根据HTTP请求的内容来返回不同的响应。以下是一个简单的示例:
```java
public class NtripServerHandler extends SimpleChannelInboundHandler<FullHttpRequest> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, FullHttpRequest request) throws Exception {
String content = "Hello NtripServer!";
FullHttpResponse response = new DefaultFullHttpResponse(HttpVersion.HTTP_1_1,
HttpResponseStatus.OK, Unpooled.copiedBuffer(content, CharsetUtil.UTF_8));
response.headers().set(HttpHeaderNames.CONTENT_TYPE, "text/plain;charset=UTF-8");
response.headers().set(HttpHeaderNames.CONTENT_LENGTH, response.content().readableBytes());
ctx.writeAndFlush(response).addListener(ChannelFutureListener.CLOSE);
}
}
```
在上面的代码中,我们解析了HTTP请求并返回了一个简单的响应。注意,我们在最后调用了`addListener(ChannelFutureListener.CLOSE)`来关闭连接。
4. 配置Spring Boot路由
最后,我们需要使用Spring Boot提供的注解来配置HTTP请求的路由。以下是一个简单的示例:
```java
@RestController
public class NtripController {
@GetMapping("/ntrip")
public String ntrip() {
return "Hello NtripServer!";
}
}
```
在上面的代码中,我们使用`@RestController`注解声明了一个控制器,并使用`@GetMapping("/ntrip")`注解来配置路由。当收到HTTP GET请求时,Spring Boot将自动调用`ntrip`方法并返回响应。
至此,我们已经完成了一个简单的Spring Boot NtripServer的实现。当然,具体的实现细节还需要根据实际业务需求进行调整和完善。
相关推荐
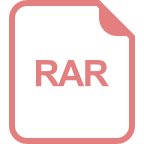
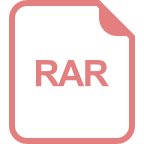














