python opencv读取文件夹里的图像旋转180度并保存
时间: 2023-10-29 09:07:18 浏览: 125
你可以按照以下步骤使用OpenCV在Python中读取文件夹中的图像,将其旋转180度并保存:
1. 导入OpenCV库
```python
import cv2
import os
```
2. 定义函数来旋转图像
```python
def rotate_image(image):
# 获取图像的宽度和高度
(h, w) = image.shape[:2]
# 计算旋转中心点的坐标
center = (w // 2, h // 2)
# 定义旋转角度为180度
angle = 180
# 通过指定旋转中心、旋转角度和缩放比例来构造旋转矩阵
M = cv2.getRotationMatrix2D(center, angle, 1.0)
# 应用旋转矩阵来旋转图像
rotated = cv2.warpAffine(image, M, (w, h))
# 返回旋转后的图像
return rotated
```
3. 遍历文件夹中的所有图像文件
```python
# 定义要读取的文件夹路径
folder_path = '/path/to/your/folder'
# 遍历文件夹中的所有图像文件
for filename in os.listdir(folder_path):
# 如果文件名以'.jpg'或'.png'结尾,则说明是图像文件
if filename.endswith('.jpg') or filename.endswith('.png'):
# 构造图像文件的完整路径
image_path = os.path.join(folder_path, filename)
# 读取图像文件
image = cv2.imread(image_path)
# 对图像进行旋转
rotated_image = rotate_image(image)
# 构造旋转后的图像文件的完整路径
rotated_image_path = os.path.join(folder_path, 'rotated_' + filename)
# 保存旋转后的图像文件
cv2.imwrite(rotated_image_path, rotated_image)
```
这样,文件夹中的所有图像文件都会被旋转180度并保存。
阅读全文
相关推荐
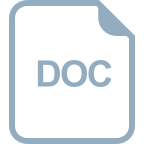
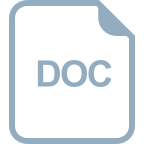
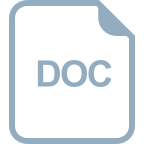
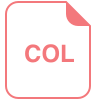
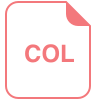
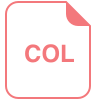
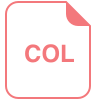
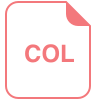
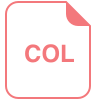

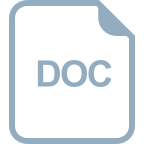
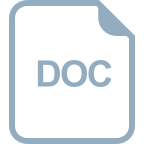
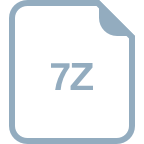
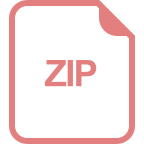
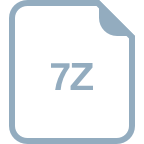