JAVA实现看门狗线程
时间: 2023-11-28 15:06:34 浏览: 133
看门狗线程是一种特殊的线程,它的主要作用是监控其他线程的运行状态,如果发现其他线程出现异常或停止运行,就会采取相应的措施,比如重新启动线程,或者记录日志等。
在Java中,可以通过继承Thread类或实现Runnable接口来创建线程,同样也可以通过继承Thread类或实现Runnable接口来创建看门狗线程。下面是一个简单的看门狗线程的实现示例:
```
public class WatchDog extends Thread {
private Thread targetThread;
private long timeout;
public WatchDog(Thread targetThread, long timeout) {
this.targetThread = targetThread;
this.timeout = timeout;
}
public void run() {
while (true) {
try {
Thread.sleep(timeout);
} catch (InterruptedException e) {
// ignore
}
if (!targetThread.isAlive()) {
System.out.println("target thread is dead, restarting...");
targetThread.start();
}
}
}
}
```
在上述代码中,WatchDog类继承了Thread类,它的构造函数需要传入一个目标线程和超时时间。在run方法中,WatchDog线程会每隔一段时间检查一次目标线程的状态,如果发现目标线程已经死亡,就会重新启动目标线程。
使用该看门狗线程可以这样实现:
```
public class Main {
public static void main(String[] args) {
Thread targetThread = new MyThread();
WatchDog watchDog = new WatchDog(targetThread, 5000);
targetThread.start();
watchDog.start();
}
}
```
在上述代码中,我们创建了一个MyThread线程作为目标线程,然后创建一个WatchDog线程来监控MyThread线程的运行状态。在main方法中,首先启动MyThread线程,然后再启动WatchDog线程,这样就可以实现看门狗线程的功能了。
阅读全文
相关推荐

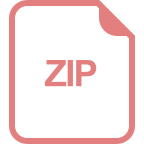
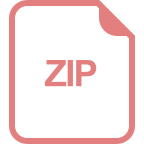
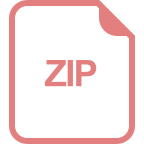
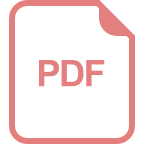
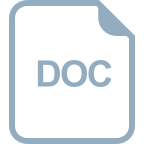
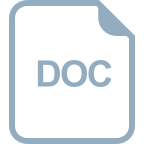
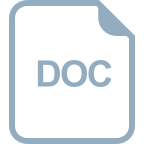
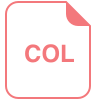




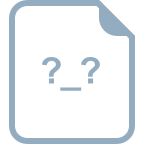