springboot实现图书管理系统
时间: 2023-04-23 14:05:40 浏览: 115
Spring Boot可以用来实现图书管理系统。可以使用Spring Boot的MVC框架来实现图书的增删改查功能,使用Spring Data JPA来管理数据库。同时,可以使用Thymeleaf模板引擎来实现前端页面的渲染,使用Bootstrap来美化页面。此外,还可以使用Spring Security来实现用户登录和权限控制。总之,Spring Boot提供了很多便利的工具和框架,可以帮助我们快速地开发出一个高效、安全、易维护的图书管理系统。
相关问题
基于springboot实现图书管理系统
基于Spring Boot实现的图书管理系统,可以为图书馆或书店提供完善的管理和服务。Spring Boot是一种轻量级的Java框架,可以快速搭建一个Web应用程序,而图书管理系统需要涉及到数据存储、业务逻辑处理、用户权限管理等多方面的功能,因此利用Spring Boot框架能够极大地简化开发流程,提高开发效率。
在图书管理系统中,需要管理书籍的基本信息,例如书名、作者、出版社、出版日期、ISBN号码等,同时还需要管理每本书籍的库存、借阅状态等信息。利用Spring Boot框架,可以使用MyBatis等持久层框架实现大量的数据处理和管理。同时,还能够基于Spring Security实现用户权限验证和管理,确保不同角色的用户能够访问和操作不同的功能。
另外,利用Spring Boot框架的特性,还可以快速构建RESTful API服务,为其他应用程序提供服务接口。图书管理系统的API接口可以使其他应用程序如移动应用程序或第三方服务通过API接口获取有关书籍的基本信息,实现更加广泛的应用。
综上所述,利用Spring Boot实现的图书管理系统不仅可以实现图书的基本管理,还可以基于现代化的Web应用程序框架构建出RESTful API服务,为其他应用程序提供服务接口。这样能够提高图书管理效率同时还能够更加方便客户获得有关书籍信息。
springboot实现图书管理系统过程例子
好的,下面是一个简单的基于Spring Boot实现的图书管理系统的例子,包含基本的增删改查功能。
1. 创建一个Spring Boot项目,引入相关依赖。
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
```
2. 创建一个图书实体类Book,使用JPA注解标识实体属性和关系。
```java
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String title;
private String author;
private String description;
// getter/setter
}
```
3. 创建一个BookRepository接口,继承JpaRepository接口,用于操作数据库。
```java
public interface BookRepository extends JpaRepository<Book, Long> {
}
```
4. 创建一个BookController类,用于处理请求和返回视图。
```java
@Controller
public class BookController {
@Autowired
private BookRepository bookRepository;
@GetMapping("/")
public String index(Model model) {
List<Book> books = bookRepository.findAll();
model.addAttribute("books", books);
return "index";
}
@GetMapping("/add")
public String addForm(Model model) {
model.addAttribute("book", new Book());
return "add";
}
@PostMapping("/add")
public String addSubmit(@ModelAttribute Book book) {
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/edit/{id}")
public String editForm(@PathVariable Long id, Model model) {
Book book = bookRepository.findById(id).orElseThrow(() -> new IllegalArgumentException("Invalid book id:" + id));
model.addAttribute("book", book);
return "edit";
}
@PostMapping("/edit/{id}")
public String editSubmit(@PathVariable Long id, @ModelAttribute Book book) {
book.setId(id);
bookRepository.save(book);
return "redirect:/";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable Long id) {
bookRepository.deleteById(id);
return "redirect:/";
}
}
```
5. 创建Thymeleaf模板,用于展示图书信息和表单。
index.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>图书管理系统</title>
</head>
<body>
<h1>图书列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>书名</th>
<th>作者</th>
<th>简介</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="book : ${books}">
<td th:text="${book.id}"></td>
<td th:text="${book.title}"></td>
<td th:text="${book.author}"></td>
<td th:text="${book.description}"></td>
<td>
<a th:href="@{/edit/{id}(id=${book.id})}">编辑</a>
<a th:href="@{/delete/{id}(id=${book.id})}">删除</a>
</td>
</tr>
</tbody>
</table>
<br>
<a th:href="@{/add}">添加图书</a>
</body>
</html>
```
add.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>添加图书</title>
</head>
<body>
<h1>添加图书</h1>
<form th:action="@{/add}" th:object="${book}" method="post">
<table>
<tr>
<td>书名:</td>
<td><input type="text" th:field="*{title}"></td>
</tr>
<tr>
<td>作者:</td>
<td><input type="text" th:field="*{author}"></td>
</tr>
<tr>
<td>简介:</td>
<td><textarea th:field="*{description}"></textarea></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="添加"></td>
</tr>
</table>
</form>
</body>
</html>
```
edit.html
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>编辑图书</title>
</head>
<body>
<h1>编辑图书</h1>
<form th:action="@{/edit/{id}(id=${book.id})}" th:object="${book}" method="post">
<table>
<tr>
<td>书名:</td>
<td><input type="text" th:field="*{title}"></td>
</tr>
<tr>
<td>作者:</td>
<td><input type="text" th:field="*{author}"></td>
</tr>
<tr>
<td>简介:</td>
<td><textarea th:field="*{description}"></textarea></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="保存"></td>
</tr>
</table>
</form>
</body>
</html>
```
6. 启动应用,访问http://localhost:8080/,可以看到图书列表,点击添加图书按钮,可以添加一本新图书,点击编辑按钮,可以修改图书信息,点击删除按钮,可以删除图书。
以上就是一个简单的基于Spring Boot实现的图书管理系统例子,希望能对你有所帮助。
阅读全文
相关推荐
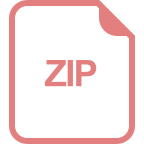
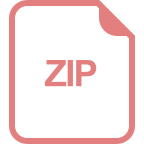

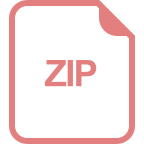
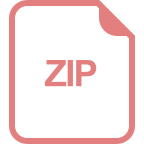
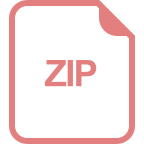
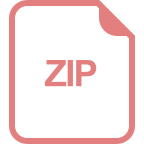
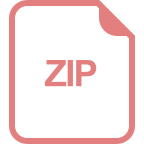
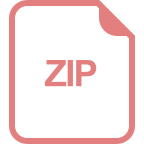
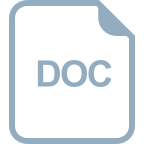




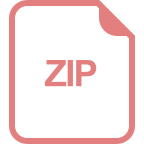
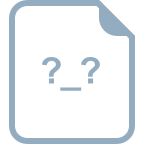