教师信息管理 (可选用结构体数组或链表完成) 。 已知教师对应的数据类型定义如下,包括:编号、部门、性别。 struct teacher{ char id[10]; char dept[30]; char sex; }; 请编写程序统计某一部门(注意:不是所有部门)的教师男女比例值。 输入信息提示:"请输入教师人数:\n" 输入信息格式:"%d" 输入信息提示:"请输入第%d个教师的编号:" 输入信息格式:"%d" 输入信息提示:"请入第%d个教师的部门:" 输入信息格式:"%d" 输入信息提示:"请入第%d个教师的性别:" 输入信息格式:"%d" 输入信息提示:"请输入需要统计的部门名字:\n" 输入信息提示:字符串 输出信息提示:"%s部门的教师男女比例值为:%d:%d" 程序运行示例: 请输入教师人数:2 请入第1个教师的编号: 00001 请入第1个教师的部门:computer technique 请入第1个教师的性别:man 请入第2个教师的编号:00002 请入第2个教师的部门:computer science 请入第2个教师的性别:woman 请输入需要统计的部门名字:computer technique (部门的教师男女比例值为:0:0
时间: 2023-06-26 18:03:21 浏览: 83
以下是使用结构体数组实现的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_TEACHERS 100
struct teacher {
char id[10];
char dept[30];
char sex;
};
int main() {
struct teacher teachers[MAX_TEACHERS];
int n, i, male_count = 0, female_count = 0;
char target_dept[30];
printf("请输入教师人数:\n");
scanf("%d", &n);
for (i = 0; i < n; i++) {
printf("请输入第%d个教师的编号:\n", i + 1);
scanf("%s", teachers[i].id);
printf("请入第%d个教师的部门:\n", i + 1);
scanf("%s", teachers[i].dept);
printf("请入第%d个教师的性别:\n", i + 1);
scanf(" %c", &teachers[i].sex);
}
printf("请输入需要统计的部门名字:\n");
scanf("%s", target_dept);
for (i = 0; i < n; i++) {
if (strcmp(teachers[i].dept, target_dept) == 0) {
if (teachers[i].sex == 'm' || teachers[i].sex == 'M') {
male_count++;
} else if (teachers[i].sex == 'f' || teachers[i].sex == 'F') {
female_count++;
}
}
}
printf("%s部门的教师男女比例值为:%d:%d", target_dept, male_count, female_count);
return 0;
}
```
以下是使用链表实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct teacher {
char id[10];
char dept[30];
char sex;
struct teacher *next;
};
struct teacher *create_teacher(char *id, char *dept, char sex) {
struct teacher *t = (struct teacher *) malloc(sizeof(struct teacher));
strcpy(t->id, id);
strcpy(t->dept, dept);
t->sex = sex;
t->next = NULL;
return t;
}
void add_teacher(struct teacher **head, struct teacher *t) {
if (*head == NULL) {
*head = t;
} else {
struct teacher *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = t;
}
}
void free_teacher_list(struct teacher *head) {
struct teacher *p = head, *q;
while (p != NULL) {
q = p->next;
free(p);
p = q;
}
}
int main() {
struct teacher *head = NULL;
int n, male_count = 0, female_count = 0;
char target_dept[30];
printf("请输入教师人数:\n");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
char id[10], dept[30], sex;
printf("请输入第%d个教师的编号:\n", i + 1);
scanf("%s", id);
printf("请入第%d个教师的部门:\n", i + 1);
scanf("%s", dept);
printf("请入第%d个教师的性别:\n", i + 1);
scanf(" %c", &sex);
struct teacher *t = create_teacher(id, dept, sex);
add_teacher(&head, t);
}
printf("请输入需要统计的部门名字:\n");
scanf("%s", target_dept);
struct teacher *p = head;
while (p != NULL) {
if (strcmp(p->dept, target_dept) == 0) {
if (p->sex == 'm' || p->sex == 'M') {
male_count++;
} else if (p->sex == 'f' || p->sex == 'F') {
female_count++;
}
}
p = p->next;
}
printf("%s部门的教师男女比例值为:%d:%d", target_dept, male_count, female_count);
free_teacher_list(head);
return 0;
}
```
阅读全文
相关推荐
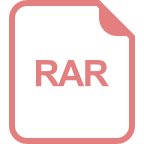

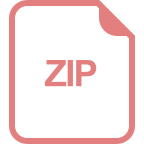

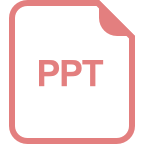
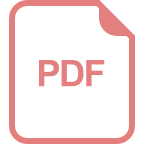
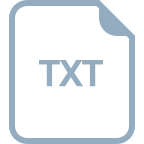
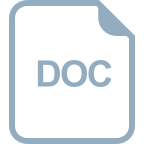
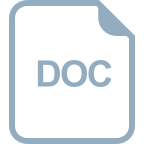
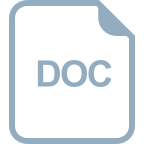
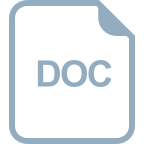
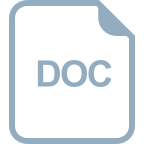
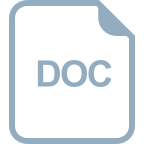
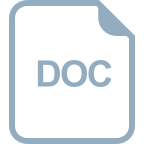
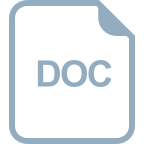