使用Java实现简单的局域网设备扫描
时间: 2024-01-24 13:03:53 浏览: 77
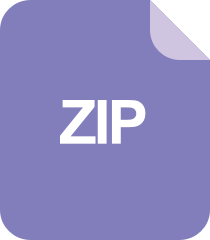
Java多线程端口快速扫描
要实现局域网设备扫描,可以使用Java中的Socket类和InetAddress类。
首先,需要获取本地IP地址,并将其转换为InetAddress类型:
```java
InetAddress myAddress = InetAddress.getLocalHost();
```
接着,可以获取子网掩码并计算出局域网IP地址范围:
```java
byte[] addressBytes = myAddress.getAddress();
int subnetMaskLength = 24; // 子网掩码长度,一般为24位
int subnetMask = 0xffffffff << (32 - subnetMaskLength);
int networkAddress = Byte.toUnsignedInt(addressBytes[0]) << 24 |
Byte.toUnsignedInt(addressBytes[1]) << 16 |
Byte.toUnsignedInt(addressBytes[2]) << 8;
int startAddress = networkAddress & subnetMask;
int endAddress = startAddress | ~subnetMask;
```
然后,可以使用Socket类来扫描局域网内的设备。对于每个IP地址,可以创建一个Socket并尝试连接到一个已知的端口(如80或443)。如果连接成功,则说明该IP地址对应的设备存在。
```java
for (int i = startAddress + 1; i < endAddress; i++) {
String ipAddress = String.format("%d.%d.%d.%d",
(i >> 24) & 0xff, (i >> 16) & 0xff, (i >> 8) & 0xff, i & 0xff);
try (Socket socket = new Socket()) {
socket.connect(new InetSocketAddress(ipAddress, 80), 1000); // 尝试连接80端口
System.out.println(ipAddress + " is up");
} catch (IOException ex) {
// 无法连接,说明设备不存在或不可达
}
}
```
完整代码如下:
```java
import java.io.IOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.Socket;
public class DeviceScanner {
public static void main(String[] args) throws IOException {
InetAddress myAddress = InetAddress.getLocalHost();
byte[] addressBytes = myAddress.getAddress();
int subnetMaskLength = 24; // 子网掩码长度,一般为24位
int subnetMask = 0xffffffff << (32 - subnetMaskLength);
int networkAddress = Byte.toUnsignedInt(addressBytes[0]) << 24 |
Byte.toUnsignedInt(addressBytes[1]) << 16 |
Byte.toUnsignedInt(addressBytes[2]) << 8;
int startAddress = networkAddress & subnetMask;
int endAddress = startAddress | ~subnetMask;
for (int i = startAddress + 1; i < endAddress; i++) {
String ipAddress = String.format("%d.%d.%d.%d",
(i >> 24) & 0xff, (i >> 16) & 0xff, (i >> 8) & 0xff, i & 0xff);
try (Socket socket = new Socket()) {
socket.connect(new InetSocketAddress(ipAddress, 80), 1000); // 尝试连接80端口
System.out.println(ipAddress + " is up");
} catch (IOException ex) {
// 无法连接,说明设备不存在或不可达
}
}
}
}
```
阅读全文
相关推荐
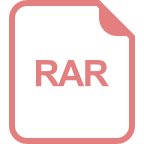
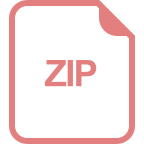














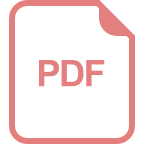