const list = ref([]);获取list的长度
时间: 2023-10-02 19:07:38 浏览: 416
可以使用 `list.value.length` 来获取 `list` 这个响应式数组的长度。由于 `list` 是一个响应式对象,因此需要使用 `.value` 属性来访问其内部的值。以下是一个示例:
```javascript
import { ref } from 'vue';
const list = ref([1, 2, 3, 4, 5]);
const length = list.value.length;
console.log(length); // 输出:5
```
在上面的示例中,我们使用 `ref` 创建了一个响应式数组 `list`,并通过 `.value` 属性获取了其内部的值。然后,我们使用 `length` 属性获取了数组的长度,并将其赋值给变量 `length`。最后,我们通过 `console.log` 输出了数组的长度。
相关问题
const optionList = ref([])
在JavaScript中,`const optionList = ref([])` 这行代码的含义取决于它出现的上下文。通常,这种语法看起来像是Vue.js框架中的一部分,具体来说是在Vue 3 Composition API中使用ref函数创建响应式引用的方式。
在这个上下文中,`ref([])` 是一个函数调用,它会返回一个对象,这个对象包含一个`.value`属性,用于存储和修改引用的数据。初始化时,这个数据是一个空数组 `[]`。`const optionList` 是这个响应式引用的名称。
这里是使用Vue 3 Composition API时,创建一个名为 `optionList` 的响应式引用并初始化为空数组的代码示例:
```javascript
import { ref } from 'vue';
const optionList = ref([]);
```
这个 `optionList` 现在是一个响应式的数据源,意味着任何对 `optionList.value` 的修改都会触发Vue组件的重新渲染。
如果你在使用Vue.js框架,并且是在编写使用Composition API的组件,那么这行代码会非常有用。使用 `ref` 创建的数据,当它们的 `.value` 属性被更新时,Vue会自动追踪这些变化,并在DOM中作出相应的更新。
如果你有更多关于Vue.js的Composition API或者其他JavaScript相关的问题,请随时提问。
优化此代码 const openKeys = ref<string[]>([]) const selectedKeys = ref<string[]>([]) const { currentMenu, currentMenuTree, currentMenuList } = storeToRefs( useLayoutStore(), ) const rootSubmenuKeys = currentMenuList.value.filter((v: any) => { if (v.type === 0) { return v.parentId } }) watch( () => currentMenu, () => { openKeys.value = [currentMenu.value?.parentId] selectedKeys.value = [currentMenu.value?.id] }, { immediate: true }, ) const router = useRouter() /** * 点击事件 * @param e 事件对象 */ const handleClick = (e: any) => { const item = currentMenuList.value.find((_) => _.id === e.key) if (item) { router.push(item.path) } } /** * SubMenu 展开/关闭的回调 * @param e 展开的openKeys */ const onOpenChange = (e: any) => { const latestOpenKey = e.find((key: any) => openKeys.value.indexOf(key) === -1) if (rootSubmenuKeys.indexOf(latestOpenKey) === -1) { openKeys.value = e } else { openKeys.value = latestOpenKey ? [latestOpenKey] : [] } }
There are a few optimizations that could be made to this code:
1. Instead of using `ref` for `openKeys` and `selectedKeys`, you can use `reactive` to make the code more concise:
```
const state = reactive({
openKeys: [],
selectedKeys: [],
})
```
2. Instead of using `storeToRefs` to convert the store state to refs, you can use the `toRefs` function, which is shorter and more concise:
```
const { currentMenu, currentMenuTree, currentMenuList } = toRefs(useLayoutStore())
```
3. Instead of using `watch` to watch the `currentMenu` state changes, you can use a computed property to update the `openKeys` and `selectedKeys` arrays:
```
const selectedMenu = computed(() => {
const item = currentMenuList.value.find((_) => _.id === currentMenu.value?.id)
return [item?.id] || []
})
const parentMenu = computed(() => {
const item = currentMenuList.value.find((_) => _.id === currentMenu.value?.parentId)
return [item?.id] || []
})
watch([selectedMenu, parentMenu], ([selected, parent]) => {
state.selectedKeys = selected
state.openKeys = parent
})
```
4. Instead of using `router.push` in the `handleClick` function, you can use the `router.push` method directly in the template:
```
<Menu.Item :key="item.id" :to="item.path">{{ item.name }}</Menu.Item>
```
5. Finally, instead of using `rootSubmenuKeys` to filter the list of menu items, you can use a computed property to filter the list of menu items based on their type:
```
const subMenuItems = computed(() => {
return currentMenuList.value.filter((v: any) => v.type === 0 && v.parentId)
})
```
By applying these optimizations, the code can be simplified and made more concise.
阅读全文
相关推荐
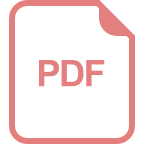
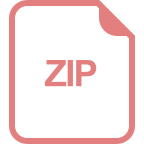













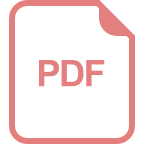
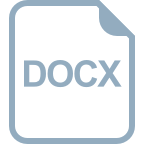