python代码 用pyqt5实现动态人脸识别界面
时间: 2023-10-04 17:11:43 浏览: 210
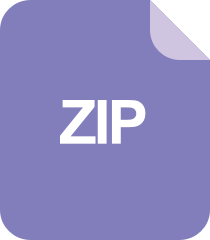
基于Python(PyQt5)实现人脸识别【100012250】

以下是一个基本的使用PyQt5实现动态人脸识别界面的代码示例。
```python
from PyQt5.QtCore import *
from PyQt5.QtGui import *
from PyQt5.QtWidgets import *
import cv2
class FaceDetectionThread(QThread):
face_detected = pyqtSignal(QImage)
def __init__(self, parent=None):
super(FaceDetectionThread, self).__init__(parent)
self.classifier = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
self.video_capture = cv2.VideoCapture(0)
def run(self):
while True:
ret, frame = self.video_capture.read()
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = self.classifier.detectMultiScale(gray, 1.3, 5)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
rgb_image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
h, w, ch = rgb_image.shape
bytes_per_line = ch * w
convert_to_qt_format = QImage(rgb_image.data, w, h, bytes_per_line, QImage.Format_RGB888)
p = convert_to_qt_format.scaled(640, 480, Qt.KeepAspectRatio)
self.face_detected.emit(p)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Face Detection')
self.image_label = QLabel(self)
self.image_label.setMinimumSize(640, 480)
self.setCentralWidget(self.image_label)
self.face_detection_thread = FaceDetectionThread()
self.face_detection_thread.face_detected.connect(self.show_image)
self.face_detection_thread.start()
def show_image(self, image):
self.image_label.setPixmap(QPixmap.fromImage(image))
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
```
在此示例中,我们使用OpenCV库进行人脸检测,并将检测到的人脸绘制到图像上。然后,我们将图像转换为Qt中的QImage格式,并在PyQt5中显示它。
在主窗口中,我们创建一个QLabel对象,并将其设置为中央控件。我们还创建一个FaceDetectionThread对象,该对象负责在后台运行人脸检测。当检测到人脸时,我们使用show_image方法将图像显示在标签上。最后,我们启动了主应用程序循环并显示主窗口。
阅读全文
相关推荐
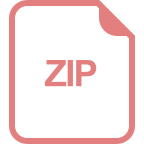

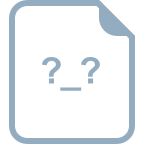
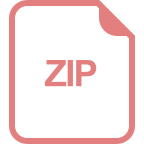
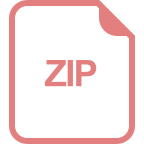
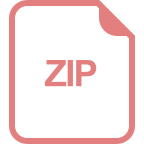
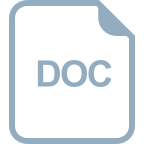




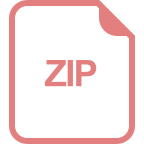
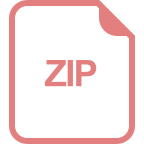
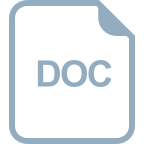
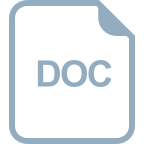

