不使用继承的方法,如何使用c++重写string::append() map list函数?
时间: 2024-03-09 22:45:24 浏览: 15
不使用继承的方法,可以使用函数重载的方法来重写这些函数,如下所示:
1. 重写string::append()
```c++
#include <iostream>
#include <string>
using namespace std;
string myAppend(const string& s1, const string& s2) {
string result = s1 + s2;
cout << "Custom append method called" << endl;
return result;
}
int main() {
string s1 = "Hello";
string s2 = " world";
string result = myAppend(s1, s2);
cout << result << endl;
return 0;
}
```
在myAppend函数中,使用重载的方式定义了一个新的append函数,实现了字符串的拼接,并添加了一些自定义的操作,这里只是简单地输出一句话。在main函数中,调用了myAppend函数,并输出了结果。
2. 重写map函数
```c++
#include <iostream>
#include <map>
using namespace std;
template<typename Key, typename Value>
void myInsert(map<Key, Value>& m, const pair<Key, Value>& p) {
m.insert(p);
cout << "Custom insert method called" << endl;
}
int main() {
map<int, string> myMap;
myInsert(myMap, make_pair(1, "Hello"));
myInsert(myMap, make_pair(2, "world"));
for (auto itr = myMap.begin(); itr != myMap.end(); itr++) {
cout << itr->first << " " << itr->second << endl;
}
return 0;
}
```
在myInsert函数中,使用重载的方式定义了一个新的insert函数,实现了键值对的插入,并添加了一些自定义的操作,这里只是简单地输出一句话。在main函数中,调用了myInsert函数,并输出了结果。
3. 重写list函数
```c++
#include <iostream>
#include <list>
using namespace std;
template<typename T>
void myPushBack(list<T>& l, const T& val) {
l.push_back(val);
cout << "Custom push_back method called" << endl;
}
int main() {
list<int> myList;
myPushBack(myList, 1);
myPushBack(myList, 2);
myPushBack(myList, 3);
for (auto itr = myList.begin(); itr != myList.end(); itr++) {
cout << *itr << " ";
}
return 0;
}
```
在myPushBack函数中,使用重载的方式定义了一个新的push_back函数,实现了元素的插入,并添加了一些自定义的操作,这里只是简单地输出一句话。在main函数中,调用了myPushBack函数,并输出了结果。
需要注意的是,在重写这些函数时,需要使用函数重载的方式来定义新的函数,并在其中实现自定义的操作。
相关推荐
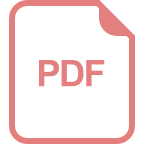
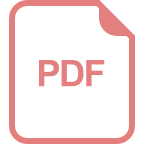
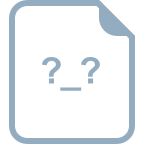














