使用c++11线程封装一个线程类
时间: 2023-12-10 09:55:06 浏览: 126
以下是一个使用C++11线程封装的线程类示例:
```c++
#include <iostream>
#include <thread>
#include <functional>
#include <atomic>
#include <vector>
class Thread {
public:
Thread() : m_thread(), m_running(false) {}
virtual ~Thread() {
if (m_running) {
stop();
}
}
void start() {
if (!m_running) {
m_running = true;
m_thread = std::thread(&Thread::run, this);
}
}
void stop() {
if (m_running) {
m_running = false;
m_thread.join();
}
}
bool isRunning() const {
return m_running;
}
protected:
virtual void run() = 0;
private:
std::thread m_thread;
std::atomic_bool m_running;
};
class MyThread : public Thread {
public:
MyThread(int id) : m_id(id) {}
protected:
void run() override {
std::cout << "Thread " << m_id << " running" << std::endl;
}
private:
int m_id;
};
int main() {
std::vector<MyThread> threads;
for (int i = 0; i < 10; ++i) {
threads.emplace_back(i);
threads.back().start();
}
for (auto& thread : threads) {
thread.stop();
}
return 0;
}
```
这个线程类有一个start方法来启动线程,一个stop方法来停止线程,一个isRunning方法来检查线程是否正在运行。这个类还有一个纯虚函数run,需要在派生类中实现。
在main函数中,我们创建了10个MyThread对象,并启动它们。然后,我们等待所有线程完成,并停止它们。
注:由于C++11线程库是跨平台的,因此可以在多个操作系统上使用该代码。
阅读全文
相关推荐
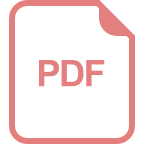
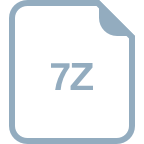
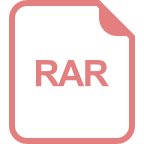
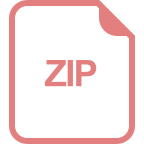
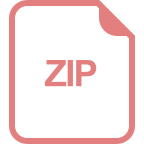
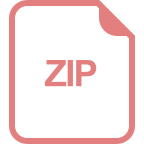
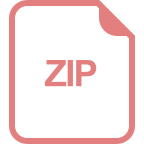
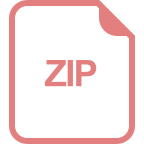
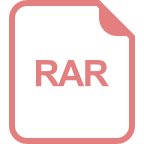
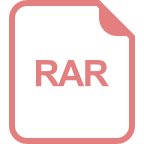
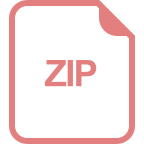
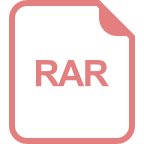
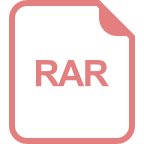
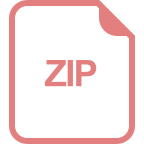
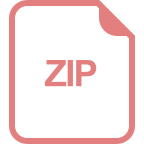
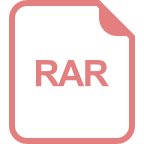
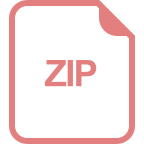
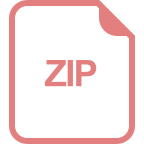