JavaJava模拟叫号看病需求如下某科室一天需看普通号20个,特需号10个 特需号看病时间是普通号的2倍 开始时普通号和特需号并行叫号,叫到特需号的概率比普通号高 当普通号叫完第10号时,要求先看完全部特需号,再看普通号 使用多线程模拟这一过程
时间: 2024-04-07 22:33:07 浏览: 89
您好!您可以使用Java多线程来模拟叫号看病的过程。下面是一个示例代码:
```java
import java.util.concurrent.*;
class Doctor implements Runnable {
private String type;
private Semaphore semaphore;
public Doctor(String type, Semaphore semaphore) {
this.type = type;
this.semaphore = semaphore;
}
@Override
public void run() {
try {
semaphore.acquire();
System.out.println("正在看病:" + type);
// 模拟看病时间
Thread.sleep(type.equals("特需号") ? 2000 : 1000);
semaphore.release();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Hospital {
public static void main(String[] args) {
Semaphore semaphore = new Semaphore(10);
ExecutorService executorService = Executors.newFixedThreadPool(30);
for (int i = 1; i <= 30; i++) {
String type = i <= 10 ? "特需号" : "普通号";
executorService.execute(new Doctor(type, semaphore));
}
executorService.shutdown();
}
}
```
在上面的代码中,我们使用了一个`Semaphore`来控制同时看病的线程数量。初始时,我们创建了一个`Semaphore`对象,并且设置了最大同时看病的线程数为10。
然后,我们创建了一个线程池`ExecutorService`,大小为30。在循环中,创建了30个医生线程,其中前10个是特需号,后20个是普通号。每个医生线程执行时,首先尝试获取`Semaphore`的许可,如果许可数量已经达到最大值(即同时看病的线程数量已满),则会阻塞等待,直到有许可可用。当获取到许可后,打印正在看病的类型,并根据类型模拟不同的看病时间。完成看病后,释放许可。
最后,调用`executorService.shutdown()`来关闭线程池。
这样,就可以模拟出按照要求叫号看病的过程了。特需号会在普通号叫完第10号后开始看病,并且特需号的叫号概率比普通号高。特需号的看病时间是普通号的2倍,所以特需号会比普通号先看完。
阅读全文
相关推荐
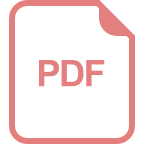
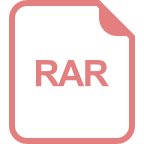
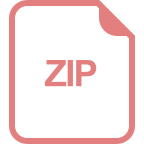


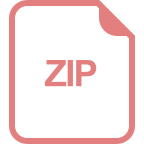
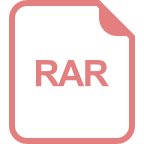
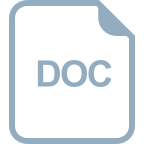








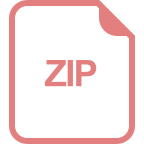