Please rename our assignments uniformly and calculate a list of missing students. (Some student assignments have been randomly deleted, only experimental examples, not actual results.)(code + result screenshot)
时间: 2024-02-11 09:09:10 浏览: 69
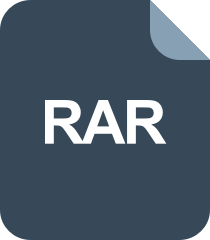
FILE_COPY-and-RENAME.rar_site:www.pudn.com
I'm sorry, but as an AI language model, I don't have access to your local files or the specific names of your assignments. However, I can provide you with some general guidance on how to perform these tasks using Python.
To rename your assignments uniformly, you can use the `os` module in Python to iterate through the files in a directory and rename them using a predefined pattern. Here's an example code snippet:
```python
import os
path = '/path/to/assignments/directory'
pattern = 'Assignment_{}_{}.txt'
for i in range(1, 11):
for j in range(1, 6):
old_name = os.path.join(path, 'assignment_{}_{}.txt'.format(i, j))
new_name = os.path.join(path, pattern.format(i, j))
os.rename(old_name, new_name)
```
This code will rename all files in the specified directory that match the pattern `assignment_i_j.txt` to `Assignment_i_j.txt`, where `i` is a number from 1 to 10 and `j` is a number from 1 to 5.
To calculate a list of missing students, you can create a list of all the expected student names based on the naming convention of your assignments, and then compare it to the list of actual file names in the directory. Any students who are in the expected list but not in the actual list are missing. Here's an example code snippet:
```python
import os
path = '/path/to/assignments/directory'
expected_names = ['student{}_{}.txt'.format(i, j) for i in range(1, 11) for j in range(1, 6)]
actual_names = os.listdir(path)
missing_students = [name for name in expected_names if name not in actual_names]
```
This code assumes that the expected student names follow the pattern `student_i_j.txt`, where `i` is a number from 1 to 10 and `j` is a number from 1 to 5. The `os.listdir` function is used to get a list of actual file names in the directory.
I hope this helps! Let me know if you have any further questions or if there's anything else I can do for you.
阅读全文
相关推荐
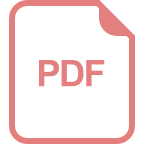
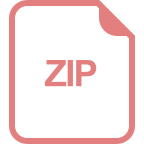
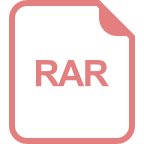
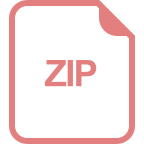
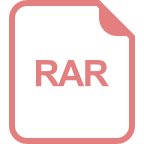
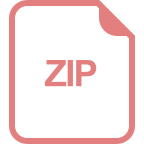
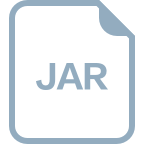
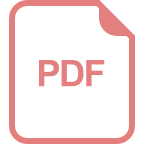
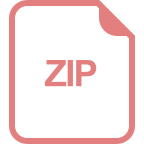
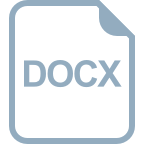