Androidstudio制作一个imageviewer且四张图片都在gridview里,点击一张图片,它会全屏展示,可以用seekbar调整它的透明度和对比度。sliding finger以切换图片。请生成详细代码
时间: 2024-05-02 21:20:38 浏览: 9
好的,我会尽力回答你的问题。以下是可以用来制作一个imageviewer并在gridview中展示四张图片的详细代码(不包括透明度和对比度的部分)。
首先,在Java文件中,我们需要创建一个继承自BaseAdapter类的适配器,用来显示图片和处理点击事件。代码如下:
```
public class ImageAdapter extends BaseAdapter {
private Context mContext;
private LayoutInflater mInflater;
private Integer[] mImageIds = { R.drawable.image1, R.drawable.image2,
R.drawable.image3, R.drawable.image4 };
public ImageAdapter(Context context) {
mContext = context;
mInflater = LayoutInflater.from(context);
}
public int getCount() {
return mImageIds.length;
}
public Object getItem(int position) {
return mImageIds[position];
}
public long getItemId(int position) {
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(R.layout.grid_item, null);
holder = new ViewHolder();
holder.image = (ImageView) convertView.findViewById(R.id.image);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
holder.image.setImageResource(mImageIds[position]);
final int pos = position;
holder.image.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent intent = new Intent(mContext, FullImageActivity.class);
intent.putExtra("id", mImageIds[pos]);
mContext.startActivity(intent);
}
});
return convertView;
}
static class ViewHolder {
ImageView image;
}
}
```
接下来,我们在res/layout文件夹中新建一个grid_item.xml文件,用来定义每个网格视图的布局。代码如下:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/image"
android:layout_width="100dp"
android:layout_height="100dp"
android:scaleType="centerCrop" />
</RelativeLayout>
```
然后,我们需要创建一个FullImageActivity类,用来显示用户点击的图片。代码如下:
```
public class FullImageActivity extends Activity {
private ImageView mImageView;
private SeekBar mSeekBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.full_image);
mImageView = (ImageView) findViewById(R.id.full_image);
mSeekBar = (SeekBar) findViewById(R.id.seek_bar);
Intent intent = getIntent();
int id = intent.getIntExtra("id", 0);
mImageView.setImageResource(id);
}
}
```
最后,在res/layout文件夹中新建一个full_image.xml文件,用来定义全屏视图的布局。代码如下:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<ImageView
android:id="@+id/full_image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter" />
<LinearLayout
android:id="@+id/slider_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="vertical" >
<SeekBar
android:id="@+id/seek_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
</RelativeLayout>
```
需要注意的是,在这里,我们只提供了展示图片和切换图片的功能。如果你需要添加更多的特性,例如透明度和对比度调整,你需要在FullImageActivity类中添加相应的代码,或者使用现有的第三方库来实现这些功能。
相关推荐
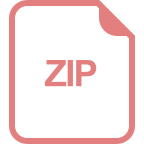
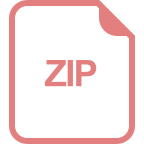














