用c 语言写 get http 调用接口,入参是user 出参是{ "code": 600, "msg": "成功", "data": null }
时间: 2023-11-05 11:57:11 浏览: 88
可以使用libcurl库来实现http请求,以下是一个使用GET方法调用接口的示例代码,入参为user:
```
#include <stdio.h>
#include <curl/curl.h>
int main(void)
{
CURL *curl;
CURLcode res;
char *url = "http://example.com/api?user=user";
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, url);
res = curl_easy_perform(curl);
if(res != CURLE_OK)
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
curl_easy_cleanup(curl);
}
return 0;
}
```
对于出参,可以使用cJSON库来解析返回的json字符串,以下是一个使用cJSON库解析json字符串并取出code、msg、data字段的示例代码:
```
#include <stdio.h>
#include <curl/curl.h>
#include "cJSON.h"
int main(void)
{
CURL *curl;
CURLcode res;
char *url = "http://example.com/api?user=user";
char *response = "";
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, url);
res = curl_easy_perform(curl);
if(res == CURLE_OK) {
response = curl_easy_escape(curl, response, 0);
cJSON *json = cJSON_Parse(response);
if (json) {
int code = cJSON_GetObjectItem(json, "code")->valueint;
char *msg = cJSON_GetObjectItem(json, "msg")->valuestring;
cJSON *data = cJSON_GetObjectItem(json, "data");
printf("code: %d\n", code);
printf("msg: %s\n", msg);
if (cJSON_IsNull(data)) {
printf("data: null\n");
} else {
// do something with data
}
cJSON_Delete(json);
}
} else {
fprintf(stderr, "curl_easy_perform() failed: %s\n",
curl_easy_strerror(res));
}
curl_easy_cleanup(curl);
}
return 0;
}
```
阅读全文
相关推荐
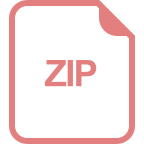
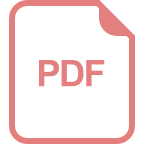

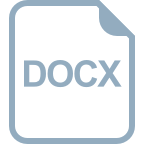
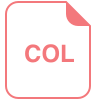
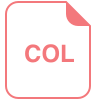
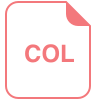
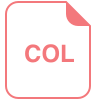
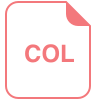
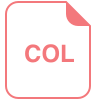
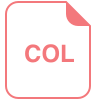
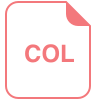
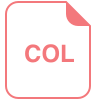
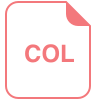
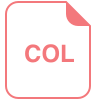
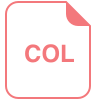
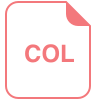
