图像定位(Python版)
时间: 2023-06-28 16:07:33 浏览: 33
图像定位是指在一张图片中找到特定目标的位置。Python中可以使用OpenCV库和PIL库实现图像定位。
下面是使用OpenCV库实现图像定位的示例代码:
```python
import cv2
# 读取原始图片
img = cv2.imread('image.jpg')
# 读取目标图片
target = cv2.imread('target.jpg')
# 获取目标图片的高度和宽度
target_h, target_w = target.shape[:2]
# 使用匹配模板函数进行定位
result = cv2.matchTemplate(img, target, cv2.TM_CCOEFF_NORMED)
# 获取最大匹配值的位置
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(result)
# 获取匹配位置
top_left = max_loc
bottom_right = (top_left[0] + target_w, top_left[1] + target_h)
# 在原始图片中绘制矩形框
cv2.rectangle(img, top_left, bottom_right, (0,0,255), 2)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
```
上述代码中,首先读取原始图片和目标图片,并获取目标图片的高度和宽度。然后使用OpenCV库提供的匹配模板函数`cv2.matchTemplate()`进行定位,最后获取匹配位置并在原始图片中绘制矩形框。
PIL库也可以实现图像定位,下面是使用PIL库实现图像定位的示例代码:
```python
from PIL import Image
import numpy as np
# 读取原始图片
img = Image.open('image.jpg')
# 读取目标图片
target = Image.open('target.jpg')
# 获取目标图片的高度和宽度
target_w, target_h = target.size
# 转换为numpy数组
img_array = np.array(img)
target_array = np.array(target)
# 使用匹配函数进行定位
result = np.zeros((img_array.shape[0]-target_h, img_array.shape[1]-target_w))
for i in range(result.shape[0]):
for j in range(result.shape[1]):
diff = img_array[i:i+target_h,j:j+target_w,:] - target_array
result[i,j] = np.sum(diff**2)
# 获取最小值的位置
min_loc = np.unravel_index(np.argmin(result), result.shape)
# 获取匹配位置
top_left = (min_loc[1], min_loc[0])
bottom_right = (top_left[0] + target_w, top_left[1] + target_h)
# 在原始图片中绘制矩形框
draw = ImageDraw.Draw(img)
draw.rectangle([top_left, bottom_right], outline='red')
# 显示结果
img.show()
```
上述代码中,首先读取原始图片和目标图片,并获取目标图片的高度和宽度。然后将图片转换为numpy数组,并使用匹配函数进行定位,最后获取匹配位置并在原始图片中绘制矩形框。需要注意的是,在PIL库中需要使用`ImageDraw`模块绘制矩形框。
相关推荐
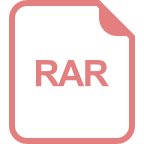











