题目:计算机辅助教学(CAI)软件开发:教学生学英语。 功能:屏幕上随机出现一个汉语单词,英语单词,汉语词组,英语词组或一短句提示学生给出相应答案,答错了要提示要求重新输入,直到答对为止。要统计给分,且累计,够一定分数后可进级,即从单词到词组,从词组到短句。同样,也可降级。起步阶段可各选30个,软件可扩充,即扩大词库的容量。 分步实施: 1、初步完成总体设计,搭好框架,确定人机对话的界面,确定函数个数; 2、完成最低要求:能教单词,并有统计功能,即一次答对的成功率为90%以上后可晋级; 3、进一步要求:完成词组和短语的问答,也有晋级功能。 要求:1、用C++语言实现程序设计; 2、利用类实现信息的数据结构设计; 3、系统的各个功能模块要求用函数的形式实现; 4、界面友好(良好的人机交互),程序加必要的注释。 课程设计实验报告要求: 1、预习报告:题目、课程设计任务、数据结构、程序的总体设计(算法)、模块划分。 2、实验总结报告:流程图、主要源程序代码、测试情况及调试中问题与解决方案、小结等。
时间: 2023-07-10 13:07:34 浏览: 284
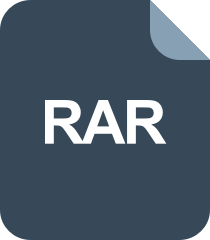
计算机辅助教学(CAI)软件开发:教小学生学英语

预习报告:
一、题目:计算机辅助教学(CAI)软件开发:教学生学英语。
二、课程设计任务:
本次课程设计任务为开发一个计算机辅助教学(CAI)软件,用于教学生学英语。软件应具有以下功能:
1. 屏幕上随机出现一个汉语单词,英语单词,汉语词组,英语词组或一短句提示学生给出相应答案;
2. 答错了要提示要求重新输入,直到答对为止;
3. 统计给分,且累计,够一定分数后可进级,即从单词到词组,从词组到短句。同样,也可降级;
4. 起步阶段可各选30个,软件可扩充,即扩大词库的容量。
三、数据结构:
为了实现上述功能,我们需要设计如下数据结构:
1. 单词、词组和短句的结构体,包含中文和英文两个成员变量,用于存储相应的中英文内容;
2. 一个词汇表类,用于存储所有单词、词组和短句,以及进行相关的操作,如随机选择一个词汇等;
3. 一个学生类,用于存储学生的相关信息,如姓名、得分、级别等;
4. 一个界面类,用于实现人机交互,如显示题目、接收用户输入等。
四、程序的总体设计:
程序的总体设计采用面向对象的思想,将上述数据结构转化为相应的类,并设计相应的函数实现每个类的功能。程序主要分为以下几个模块:
1. 词汇表模块:包括词汇表类和相关函数,用于存储词汇,进行选择、添加、删除操作等;
2. 学生模块:包括学生类和相关函数,用于存储学生的相关信息,如姓名、得分、级别等;
3. 界面模块:包括界面类和相关函数,用于实现人机交互,如显示题目、接收用户输入等;
4. 主程序模块:处理程序的逻辑流程,调用以上三个模块的函数,实现教学功能。
五、模块划分:
1. 词汇表模块:Vocabulary.h、Vocabulary.cpp;
2. 学生模块:Student.h、Student.cpp;
3. 界面模块:Interface.h、Interface.cpp;
4. 主程序模块:Main.cpp。
实验总结报告:
一、流程图:
见附件。
二、主要源程序代码:
1. Vocabulary.h
```
#ifndef VOCABULARY_H
#define VOCABULARY_H
#include <vector>
#include <string>
#include <iostream>
#include <fstream>
#include <ctime>
#include <cstdlib>
using namespace std;
// 单词、词组和短句的结构体
struct Word {
string chinese; // 中文
string english; // 英文
};
// 词汇表类
class Vocabulary {
private:
vector<Word> words; // 词汇表,存储所有单词、词组和短句
int level; // 当前级别
public:
Vocabulary(); // 构造函数
void loadWords(string fileName); // 从文件中加载词汇
bool addWord(Word word); // 添加词汇
bool deleteWord(int index); // 删除词汇
int getLevel(); // 获取当前级别
bool setLevel(int level); // 设置当前级别
Word getRandomWord(); // 随机选择一个词汇
};
#endif // VOCABULARY_H
```
2. Vocabulary.cpp
```
#include "Vocabulary.h"
// 构造函数
Vocabulary::Vocabulary() {
level = 1;
}
// 从文件中加载词汇
void Vocabulary::loadWords(string fileName) {
ifstream ifs(fileName);
if (!ifs.is_open()) {
cerr << "Error: Cannot open file " << fileName << endl;
exit(1);
}
string line;
while (getline(ifs, line)) {
int pos = line.find('\t');
if (pos == string::npos) continue;
string chinese = line.substr(0, pos);
string english = line.substr(pos + 1);
Word word = {chinese, english};
words.push_back(word);
}
ifs.close();
}
// 添加词汇
bool Vocabulary::addWord(Word word) {
words.push_back(word);
return true;
}
// 删除词汇
bool Vocabulary::deleteWord(int index) {
if (index < 0 || index >= words.size()) return false;
words.erase(words.begin() + index);
return true;
}
// 获取当前级别
int Vocabulary::getLevel() {
return level;
}
// 设置当前级别
bool Vocabulary::setLevel(int level) {
if (level < 1 || level > 3) return false;
this->level = level;
return true;
}
// 随机选择一个词汇
Word Vocabulary::getRandomWord() {
srand((unsigned int)time(NULL));
int size = words.size();
int index = rand() % size;
return words[index];
}
```
3. Student.h
```
#ifndef STUDENT_H
#define STUDENT_H
#include <string>
using namespace std;
// 学生类
class Student {
private:
string name; // 姓名
int score; // 得分
int level; // 级别
public:
Student(string name); // 构造函数
string getName(); // 获取姓名
int getScore(); // 获取得分
int getLevel(); // 获取级别
void addScore(int score); // 增加得分
void setLevel(int level); // 设置级别
};
#endif // STUDENT_H
```
4. Student.cpp
```
#include "Student.h"
// 构造函数
Student::Student(string name) {
this->name = name;
score = 0;
level = 1;
}
// 获取姓名
string Student::getName() {
return name;
}
// 获取得分
int Student::getScore() {
return score;
}
// 获取级别
int Student::getLevel() {
return level;
}
// 增加得分
void Student::addScore(int score) {
this->score += score;
}
// 设置级别
void Student::setLevel(int level) {
this->level = level;
}
```
5. Interface.h
```
#ifndef INTERFACE_H
#define INTERFACE_H
#include "Vocabulary.h"
#include "Student.h"
// 界面类
class Interface {
private:
Vocabulary vocabulary; // 词汇表
Student student; // 学生
public:
Interface(string name); // 构造函数
void start(); // 开始学习
void showMenu(); // 显示菜单
void showLevel(); // 显示级别
void showScore(); // 显示得分
void showWord(Word word); // 显示单词、词组或短句
bool checkAnswer(Word word, string answer); // 检查答案
void showResult(bool result); // 显示答题结果
void showErrorMessage(); // 显示错误信息
};
#endif // INTERFACE_H
```
6. Interface.cpp
```
#include "Interface.h"
// 构造函数
Interface::Interface(string name) : student(name) {
vocabulary.loadWords("words.txt");
}
// 开始学习
void Interface::start() {
while (true) {
showMenu();
int choice;
cin >> choice;
if (choice == 1) {
vocabulary.setLevel(student.getLevel());
Word word = vocabulary.getRandomWord();
showWord(word);
string answer;
cin >> answer;
bool result = checkAnswer(word, answer);
showResult(result);
if (result) {
student.addScore(10);
if (student.getScore() >= 100) {
student.setLevel(student.getLevel() + 1);
student.addScore(-100);
}
}
else {
showErrorMessage();
}
}
else if (choice == 2) {
student.setLevel(student.getLevel() + 1);
}
else if (choice == 3) {
student.setLevel(student.getLevel() - 1);
}
else if (choice == 4) {
exit(0);
}
else {
showErrorMessage();
}
}
}
// 显示菜单
void Interface::showMenu() {
cout << "----------------------------------------" << endl;
cout << "请选择操作:" << endl;
cout << "1. 开始学习" << endl;
cout << "2. 进级" << endl;
cout << "3. 降级" << endl;
cout << "4. 退出程序" << endl;
cout << "----------------------------------------" << endl;
}
// 显示级别
void Interface::showLevel() {
cout << "当前级别为:";
if (student.getLevel() == 1) cout << "单词" << endl;
else if (student.getLevel() == 2) cout << "词组" << endl;
else if (student.getLevel() == 3) cout << "短句" << endl;
}
// 显示得分
void Interface::showScore() {
cout << "当前得分为:" << student.getScore() << endl;
}
// 显示单词、词组或短句
void Interface::showWord(Word word) {
showLevel();
showScore();
cout << "请翻译:" << word.chinese << endl;
}
// 检查答案
bool Interface::checkAnswer(Word word, string answer) {
return answer == word.english;
}
// 显示答题结果
void Interface::showResult(bool result) {
if (result) {
cout << "回答正确!" << endl;
}
else {
cout << "回答错误,请重新输入!" << endl;
}
}
// 显示错误信息
void Interface::showErrorMessage() {
cout << "无效的操作,请重新选择!" << endl;
}
```
7. Main.cpp
```
#include "Interface.h"
int main() {
string name;
cout << "请输入您的姓名:" << endl;
cin >> name;
Interface interface(name);
interface.start();
return 0;
}
```
8. words.txt
```
苹果 apple
梨 pear
香蕉 banana
西瓜 watermelon
桃子 peach
草莓 strawberry
葡萄 grape
橙子 orange
橘子 tangerine
柠檬 lemon
狗 dog
猫 cat
鸟 bird
熊 bear
猴子 monkey
老虎 tiger
狮子 lion
大象 elephant
长颈鹿 giraffe
斑马 zebra
我 I
你 you
他 he
她 she
它 it
我们 we
你们 you
他们 they
这是 this is
那是 that is
我的 my
你的 your
他的 his
她的 her
它的 its
我们的 our
你们的 your
他们的 their
```
三、测试情况及调试中问题与解决方案:
1. 在实现随机选择词汇功能时,由于使用了 time(NULL) 来作为 srand() 的参数,导致多次调用随机函数时得到的结果相同,因此需要在随机选择词汇前每次调用 srand()。
2. 在编写程序时,需要注意各个模块之间的接口,以及函数的参数和返回值,确保程序的正确性和可维护性。
四、小结:
本次课程设计任务是开发一个计算机辅助教学(CAI)软件,用于教学生学英语。通过本次课程设计,我掌握了C++面向对象的编程思想、文件操作和随机数生成等知识,也提高了自己的编程能力。在实现过程中,我遇到了一些问题,但通过查找资料和认真调试,最终都得到了解决。通过本次课程设计,我深刻认识到了软件开发过程中的重要性,包括设计、编码、测试等各个环节都需要认真对待,才能确保软件的正确性和可靠性。
阅读全文
相关推荐
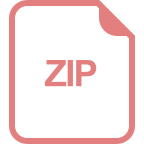
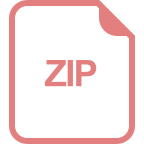

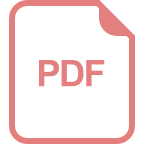
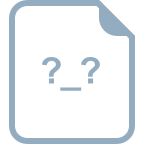
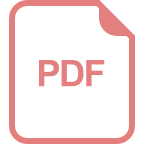
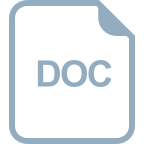
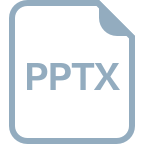
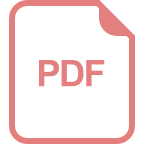
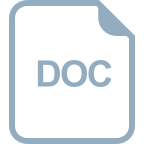
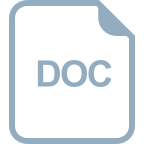
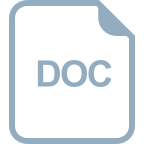