遗传算法python实例
时间: 2023-10-27 21:05:23 浏览: 55
以下是一个简单的遗传算法Python实现的示例代码:
```python
import random
# 定义目标函数
def fitness_function(chromosome):
x = chromosome[0]
y = chromosome[1]
return x**2 + y**2
# 初始化种群
def initial_population(population_size, chromosome_length):
population = []
for i in range(population_size):
chromosome = []
for j in range(chromosome_length):
chromosome.append(random.uniform(-10, 10))
population.append(chromosome)
return population
# 选择操作(轮盘赌算法)
def selection(population, fitness_values):
total_fitness = sum(fitness_values)
probabilities = [fitness / total_fitness for fitness in fitness_values]
selected_indices = []
for i in range(len(population)):
pick = random.uniform(0, 1)
current = 0
for j in range(len(population)):
current += probabilities[j]
if current > pick:
selected_indices.append(j)
break
selected_population = [population[i] for i in selected_indices]
return selected_population
# 交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异操作
def mutation(chromosome, mutation_rate):
for i in range(len(chromosome)):
if random.uniform(0, 1) < mutation_rate:
chromosome[i] += random.uniform(-1, 1)
return chromosome
# 主程序
population_size = 50
chromosome_length = 2
mutation_rate = 0.1
generations = 50
population = initial_population(population_size, chromosome_length)
for generation in range(generations):
fitness_values = [fitness_function(chromosome) for chromosome in population]
selected_population = selection(population, fitness_values)
new_population = []
while len(new_population) < population_size:
parent1, parent2 = random.sample(selected_population, 2)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
new_population.append(child1)
new_population.append(child2)
population = new_population
best_chromosome = min(population, key=fitness_function)
best_fitness = fitness_function(best_chromosome)
print("Best solution found: ", best_chromosome)
print("Best fitness found: ", best_fitness)
```
该示例代码实现了一个简单的遗传算法来最小化二元函数 $x^2 + y^2$。在主程序中,我们首先初始化种群,然后进行一系列进化操作,包括选择、交叉和变异。最后,我们找到最小化目标函数的最佳解并输出结果。
请注意,这只是一个基本的示例代码,如果要使用遗传算法解决更复杂的问题,需要根据具体情况进行调整和优化。
阅读全文
相关推荐
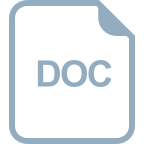
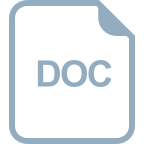
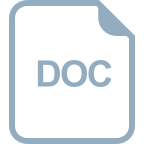
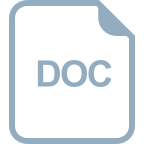
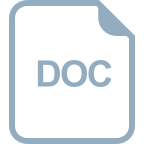
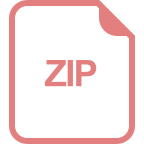
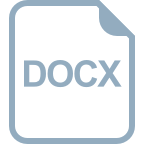
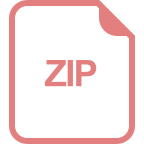
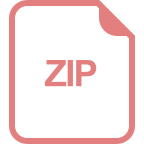
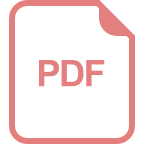