用qt编写一个随机点名的程序 要求用户可以自己添加名字 选择每次抽选的人数 不用保证上次被抽到的人下次还被抽到
时间: 2024-05-13 16:15:04 浏览: 200
以下是一个简单的随机点名程序的Qt代码示例,其中包括添加名字、选择抽选人数、随机抽选等功能:
```c++
#include <QtWidgets>
class RandomPicker : public QWidget
{
public:
RandomPicker(QWidget *parent = nullptr) : QWidget(parent)
{
// 初始化界面元素
nameLabel = new QLabel("添加名字:");
nameEdit = new QLineEdit;
addButton = new QPushButton("添加");
removeButton = new QPushButton("移除");
listWidget = new QListWidget;
listWidget->setSelectionMode(QAbstractItemView::MultiSelection);
numberLabel = new QLabel("选择抽选人数:");
numberSpin = new QSpinBox;
numberSpin->setMinimum(1);
numberSpin->setMaximum(10);
numberSpin->setValue(1);
pickButton = new QPushButton("抽选");
resultLabel = new QLabel;
// 布局
QGridLayout *layout = new QGridLayout;
layout->addWidget(nameLabel, 0, 0);
layout->addWidget(nameEdit, 0, 1);
layout->addWidget(addButton, 0, 2);
layout->addWidget(removeButton, 0, 3);
layout->addWidget(listWidget, 1, 0, 1, 4);
layout->addWidget(numberLabel, 2, 0);
layout->addWidget(numberSpin, 2, 1);
layout->addWidget(pickButton, 2, 2);
layout->addWidget(resultLabel, 2, 3);
setLayout(layout);
// 信号槽连接
connect(addButton, &QPushButton::clicked, this, &RandomPicker::addName);
connect(removeButton, &QPushButton::clicked, this, &RandomPicker::removeName);
connect(pickButton, &QPushButton::clicked, this, &RandomPicker::pickNames);
}
private:
QLabel *nameLabel;
QLineEdit *nameEdit;
QPushButton *addButton;
QPushButton *removeButton;
QListWidget *listWidget;
QLabel *numberLabel;
QSpinBox *numberSpin;
QPushButton *pickButton;
QLabel *resultLabel;
QStringList names;
void addName()
{
QString name = nameEdit->text().trimmed();
if (!name.isEmpty() && !names.contains(name)) {
names.append(name);
listWidget->addItem(name);
nameEdit->clear();
}
}
void removeName()
{
QList<QListWidgetItem*> selectedItems = listWidget->selectedItems();
foreach(QListWidgetItem *item, selectedItems) {
int row = listWidget->row(item);
listWidget->takeItem(row);
names.removeAt(row);
delete item;
}
}
void pickNames()
{
int n = numberSpin->value();
QStringList pickedNames;
for (int i = 0; i < n; i++) {
int index = qrand() % names.size();
pickedNames.append(names.at(index));
}
resultLabel->setText(pickedNames.join(", "));
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
qsrand(QTime::currentTime().msec()); // 初始化随机数生成器
RandomPicker picker;
picker.show();
return app.exec();
}
```
该程序界面主要有以下几个元素:
1. 添加名字的标签、文本框和添加按钮
2. 列表框,显示已添加的名字,支持多选删除
3. 选择抽选人数的标签和数字框
4. 抽选按钮和显示结果的标签
程序实现的主要逻辑如下:
1. 用户在文本框中输入名字,点击添加按钮,将名字添加到列表框中
2. 用户选中一个或多个名字,点击移除按钮,将这些名字从列表框中删除
3. 用户选择抽选人数,点击抽选按钮,随机抽选指定数量的名字,并在结果标签中显示这些名字
阅读全文
相关推荐
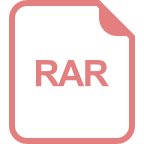













