你能给我写一封Android studio的学生信息管理系统吗
时间: 2024-03-17 16:46:48 浏览: 27
当然可以!不过在这里我只能给你提供一些基本的思路和代码示例,具体实现还需要你自己完成。以下是一些关键点:
1. UI设计:你可以使用Android Studio自带的UI设计工具或者自己手动编写XML布局文件来创建你的界面,包括主页面、添加页面、修改页面、删除页面等等。
2. 数据库设计:你需要设计一个数据库来存储学生的信息,包括姓名、学号、性别、年龄、专业等字段。你可以使用SQLite或者其他数据库来实现。
3. 数据库操作:你需要编写一些操作数据库的代码,包括增删改查等操作。以下是一个SQLite的示例:
```
public void addStudent(Student student) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(KEY_NAME, student.getName());
values.put(KEY_ID, student.getId());
values.put(KEY_GENDER, student.getGender());
values.put(KEY_AGE, student.getAge());
values.put(KEY_MAJOR, student.getMajor());
db.insert(TABLE_STUDENTS, null, values);
db.close();
}
public Student getStudent(int id) {
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = db.query(TABLE_STUDENTS, new String[] { KEY_ID,
KEY_NAME, KEY_GENDER, KEY_AGE, KEY_MAJOR }, KEY_ID + "=?",
new String[] { String.valueOf(id) }, null, null, null, null);
if (cursor != null)
cursor.moveToFirst();
Student student = new Student(cursor.getString(1), Integer.parseInt(cursor.getString(0)),
cursor.getString(2), Integer.parseInt(cursor.getString(3)), cursor.getString(4));
return student;
}
public List<Student> getAllStudents() {
List<Student> studentList = new ArrayList<Student>();
String selectQuery = "SELECT * FROM " + TABLE_STUDENTS;
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery(selectQuery, null);
if (cursor.moveToFirst()) {
do {
Student student = new Student();
student.setId(Integer.parseInt(cursor.getString(0)));
student.setName(cursor.getString(1));
student.setGender(cursor.getString(2));
student.setAge(Integer.parseInt(cursor.getString(3)));
student.setMajor(cursor.getString(4));
studentList.add(student);
} while (cursor.moveToNext());
}
return studentList;
}
public int updateStudent(Student student) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(KEY_NAME, student.getName());
values.put(KEY_GENDER, student.getGender());
values.put(KEY_AGE, student.getAge());
values.put(KEY_MAJOR, student.getMajor());
return db.update(TABLE_STUDENTS, values, KEY_ID + " = ?",
new String[] { String.valueOf(student.getId()) });
}
public void deleteStudent(Student student) {
SQLiteDatabase db = this.getWritableDatabase();
db.delete(TABLE_STUDENTS, KEY_ID + " = ?",
new String[] { String.valueOf(student.getId()) });
db.close();
}
```
4. 逻辑实现:你需要编写一些逻辑代码来实现添加、查询、修改和删除学生信息的功能。以下是一个示例:
```
public class MainActivity extends AppCompatActivity {
private EditText inputName, inputId, inputGender, inputAge, inputMajor;
private Button btnAdd, btnDelete, btnUpdate, btnGetAll;
private ListView listView;
private DatabaseHandler db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
inputName = (EditText) findViewById(R.id.input_name);
inputId = (EditText) findViewById(R.id.input_id);
inputGender = (EditText) findViewById(R.id.input_gender);
inputAge = (EditText) findViewById(R.id.input_age);
inputMajor = (EditText) findViewById(R.id.input_major);
btnAdd = (Button) findViewById(R.id.btn_add);
btnDelete = (Button) findViewById(R.id.btn_delete);
btnUpdate = (Button) findViewById(R.id.btn_update);
btnGetAll = (Button) findViewById(R.id.btn_get_all);
listView = (ListView) findViewById(R.id.list_view);
db = new DatabaseHandler(this);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String name = inputName.getText().toString();
int id = Integer.parseInt(inputId.getText().toString());
String gender = inputGender.getText().toString();
int age = Integer.parseInt(inputAge.getText().toString());
String major = inputMajor.getText().toString();
Student student = new Student(name, id, gender, age, major);
db.addStudent(student);
inputName.setText("");
inputId.setText("");
inputGender.setText("");
inputAge.setText("");
inputMajor.setText("");
Toast.makeText(MainActivity.this, "Student added", Toast.LENGTH_SHORT).show();
}
});
btnDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
int id = Integer.parseInt(inputId.getText().toString());
Student student = db.getStudent(id);
db.deleteStudent(student);
inputName.setText("");
inputId.setText("");
inputGender.setText("");
inputAge.setText("");
inputMajor.setText("");
Toast.makeText(MainActivity.this, "Student deleted", Toast.LENGTH_SHORT).show();
}
});
btnUpdate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String name = inputName.getText().toString();
int id = Integer.parseInt(inputId.getText().toString());
String gender = inputGender.getText().toString();
int age = Integer.parseInt(inputAge.getText().toString());
String major = inputMajor.getText().toString();
Student student = new Student(name, id, gender, age, major);
db.updateStudent(student);
inputName.setText("");
inputId.setText("");
inputGender.setText("");
inputAge.setText("");
inputMajor.setText("");
Toast.makeText(MainActivity.this, "Student updated", Toast.LENGTH_SHORT).show();
}
});
btnGetAll.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
List<Student> studentList = db.getAllStudents();
ArrayAdapter<Student> adapter = new ArrayAdapter<Student>(MainActivity.this,
android.R.layout.simple_list_item_1, studentList);
listView.setAdapter(adapter);
}
});
}
}
```
这些代码示例只是一个简单的学生信息管理系统的骨架,你需要根据自己的需求进行修改和完善。希望这些信息对你有所帮助!
相关推荐
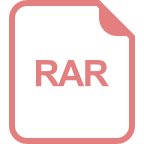
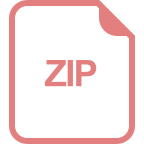















