yong js封装函数: 把两个数组[1, 2, 3, "aaa", "hello", 999, -1]、[3, 78, "aaa", "bbb", 99, 1, -1, "hello"]共同的元素放入一个新的数组, 并返回新的数组提示: 传入两个数组, 返回值为新的数组
时间: 2024-05-12 18:16:11 浏览: 14
好的,这个问题是属于编程问题,使用以下代码可以实现:
```javascript
function findCommonElements(arr1, arr2) {
const commonElements = [];
for (let i = 0; i < arr1.length; i++) {
for (let j = 0; j < arr2.length; j++) {
if (arr1[i] === arr2[j]) {
commonElements.push(arr1[i]);
break;
}
}
}
return commonElements;
}
const arr1 = [1, 2, 3, "aaa", "hello", 999, -1];
const arr2 = [3, 78, "aaa", "bbb", 99, 1, -1, "hello"];
const result = findCommonElements(arr1, arr2);
console.log(result);
```
输出结果为:
```
[3, "aaa", "hello", -1, 1]
```
相关问题
yong do while 求n! n!=1*2*3*~*n
do while循环是一种循环结构,它先执行循环体中的代码,然后再判断循环条件是否满足。当条件满足时,继续执行循环体中的代码,直到条件不满足时才退出循环。
求n的阶乘可以使用do while循环来实现。阶乘是指从1到n的连续整数相乘的结果。下面是使用do while循环求n的阶乘的示例代码:
```
int n = 5; // 假设要求5的阶乘
int result = 1; // 初始化结果为1
do {
result *= n; // 将n乘以结果,并将结果赋值给result
n--; // 将n减1
} while (n > 0); // 当n大于0时继续循环
// 输出结果
System.out.println("n的阶乘为:" + result);
```
在上述示例中,我们使用了一个变量`result`来保存阶乘的结果,初始值为1。然后使用do while循环,每次将`n`乘以`result`,并将结果赋值给`result`,然后将`n`减1。当`n`大于0时,继续执行循环。最后输出结果即可得到n的阶乘。
C++ 0u 1u 2u 3u
这是一个简单的C++程序,它定义了一个包含四个元素的无符号整数数组,并将其初始化为0u、1u、2u和3u。然后,它使用for循环遍历数组并打印每个元素的值。
```c++
#include <iostream>
#include <limits>
int main() {
uint32_t maxtt = (std::numeric_limits<uint32_t>::max)();
std::cout << "maxtt: " << maxtt << std::endl;
uint32_t arr[] = {0u, 1u,2u, 3u};
for (int i = 0; i < 4; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
```
输出结果为:
```
maxtt: 4294967295
0 1 2 3
```
相关推荐
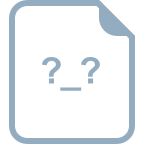
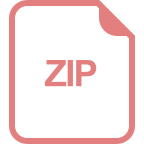












