利用给定的一组坐标,实现三次Hermite插值曲线绘制。该曲线绘制做成一个函数形式
时间: 2023-09-26 18:06:42 浏览: 86
### 回答1:
好的,我可以为您提供这方面的帮助。以下是一个实现三次Hermite插值曲线绘制的函数示例(使用Python语言):
```python
import numpy as np
import matplotlib.pyplot as plt
def hermite_interpolation(x, y, m):
n = len(x)
A = np.zeros((2*n, 2*n))
B = np.zeros((2*n, 1))
for i in range(n):
A[2*i, :] = np.array([x[i]**3, x[i]**2, x[i], 1, 0, 0, 0, 0])
A[2*i+1, :] = np.array([0, 0, 0, 0, x[i]**3, x[i]**2, x[i], 1])
B[2*i] = y[i]
B[2*i+1] = m[i]
if i > 0:
A[2*i, 2*i-2:2*i] = np.array([3*x[i-1]**2, 2*x[i-1], 1, 0])
A[2*i+1, 2*i-2:2*i] = np.array([0, 0, 0, 1])
if i < n-1:
A[2*i, 2*i+2:2*(i+1)] = np.array([-3*x[i+1]**2, -2*x[i+1], -1, 0])
A[2*i+1, 2*i+2:2*(i+1)] = np.array([0, 0, 0, -1])
c = np.linalg.solve(A, B)
return c
def hermite_curve(c, x, h=0.01):
t = np.arange(x[0], x[-1]+h, h)
y = np.zeros_like(t)
for i in range(len(x)-1):
mask = np.logical_and(t >= x[i], t < x[i+1])
xx = t[mask] - x[i]
yy = c[2*i]*xx**3 + c[2*i+1]*xx**2 + c[2*i+2]*xx + c[2*i+3]
y[mask] = yy
return t, y
# 示例坐标点
x = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
y = np.array([-2.0, 0.0, 3.0, 1.0, 2.0])
m = np.array([0.0, -3.0, 4.0, 1.0, -4.0])
# 计算插值曲线
c = hermite_interpolation(x, y, m)
t, curve = hermite_curve(c, x)
# 绘制插值曲线
plt.plot(x, y, 'ro', t, curve)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Hermite Interpolation')
plt.show()
```
请注意,这个函数实现的是三次Hermite插值曲线绘制,在使用时需要提供坐标点(x,y)和斜率(m)。函数会返回绘制的曲线数据(t,curve)供绘图使用。
希望这个函数对您有所帮助!
### 回答2:
三次Hermite插值是一种用于在给定一组坐标的情况下生成平滑曲线的方法。该方法利用给定的坐标和每个坐标点的切线信息来计算曲线上的点。
要实现三次Hermite插值曲线绘制,我们可以写一个函数。这个函数接受一个坐标的数组和相应的切线信息数组作为输入,并返回曲线上的点坐标。
首先,我们需要知道三次Hermite曲线的参数方程。给定一个坐标点(x, y)和相应的切线信息(dx, dy),我们可以使用以下方程来计算曲线上的点(x(t), y(t)):
x(t) = (2t^3 - 3t^2 + 1)x + (t^3 - 2t^2 + t)dx
y(t) = (2t^3 - 3t^2 + 1)y + (t^3 - 2t^2 + t)dy
在函数中,我们可以使用一个循环来生成曲线上的点。循环的迭代变量t从0到1,可以根据需要进行细分(如0.01)。
在每次迭代中,我们可以使用上述方程计算每个t对应的曲线上的点坐标。最后,将这些点坐标保存在一个新的数组中并返回。
以下是一个实现这个函数的示例代码:
```python
def hermite_interpolation(coordinates, tangents):
curve_points = []
for t in range(0, 101):
t = t / 100.0
x = (2*t**3 - 3*t**2 + 1) * coordinates[0][0] + (t**3 - 2*t**2 + t) * tangents[0][0]
y = (2*t**3 - 3*t**2 + 1) * coordinates[0][1] + (t**3 - 2*t**2 + t) * tangents[0][1]
curve_points.append((x, y))
return curve_points
```
在这个示例中,`coordinates`参数是一个带有坐标点的数组,`tangents`参数是一个带有相应切线信息的数组。最后,函数返回一个包含曲线上点坐标的数组`curve_points`。
利用以上函数,我们可以将给定的坐标和切线信息传递给函数,然后将返回的曲线上的点坐标用于绘制三次Hermite插值曲线。
### 回答3:
要实现三次Hermite插值曲线绘制,首先需要给定一组坐标。假设我们有四个坐标点:P0、P1、T0和T1,其中P0和P1为插值曲线经过的起始和结束点,而T0和T1则是起始和结束点的切线向量。
三次Hermite插值曲线的函数形式为:
H(t) = (2t^3 - 3t^2 + 1)P0 + (t^3 - 2t^2 + t)T0 + (-2t^3 + 3t^2)P1 + (t^3 - t^2)T1
其中t是参数,范围为[0, 1],表示曲线上的点从起始点到结束点的位置。
绘制三次Hermite插值曲线的函数可以如下所示:
```python
import numpy as np
import matplotlib.pyplot as plt
def hermite_interpolation(P0, P1, T0, T1, num_points):
t = np.linspace(0, 1, num_points)
H = (2*t**3 - 3*t**2 + 1)*P0 + (t**3 - 2*t**2 + t)*T0 + (-2*t**3 + 3*t**2)*P1 + (t**3 - t**2)*T1
return H
# 给定一组坐标点
P0 = np.array([1, 2])
P1 = np.array([3, 4])
T0 = np.array([5, 6])
T1 = np.array([7, 8])
# 调用函数绘制曲线
curve_points = hermite_interpolation(P0, P1, T0, T1, 100)
# 绘制起始点、结束点和控制点
plt.scatter([P0[0], P1[0]], [P0[1], P1[1]], color='red', label='Control Points')
plt.scatter([T0[0], T1[0]], [T0[1], T1[1]], color='blue', label='Tangent Vectors')
# 绘制插值曲线
plt.plot(curve_points[:, 0], curve_points[:, 1], color='green', label='Hermite Curve')
plt.legend()
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Hermite Interpolation')
plt.show()
```
以上代码使用numpy实现了三次Hermite插值曲线的绘制。首先根据给定的参数计算出曲线上的点,然后使用matplotlib库将起始点、结束点和控制点标出,最后绘制出插值曲线。通过调整给定坐标点的值可以得到不同的曲线形状。
相关推荐
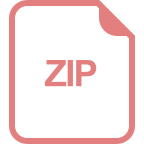
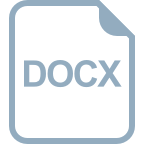
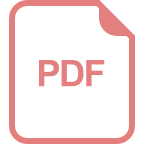
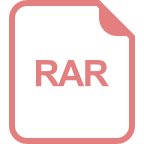
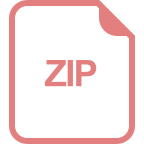
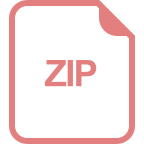
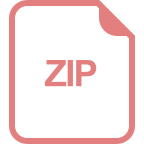
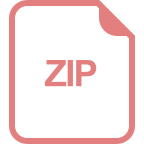
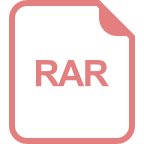
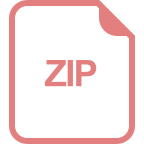
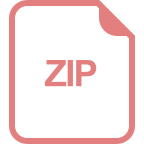
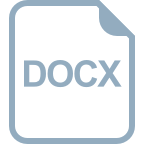
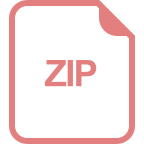