vite+elementPlus+vue3+jsplumb+pinia+sortable实现完整的流程图
时间: 2023-09-02 16:14:07 浏览: 196
要实现完整的流程图,您可以使用以下技术和工具:
1. Vue 3:Vue 3 是一个优秀的 JavaScript 框架,可以使您的应用程序更加可维护和易于开发。它具有响应式数据绑定、组件化开发、虚拟 DOM 等特性。
2. Vite:Vite 是一个快速的开发工具,可帮助您更快地构建 Web 应用程序。它可以快速地启动开发服务器,支持热模块替换和快速构建。
3. Element Plus:Element Plus 是一组基于 Vue 3 的 UI 组件库,可以帮助您快速构建漂亮的界面。
4. Jsplumb:Jsplumb 是一个流程图库,可以帮助您构建复杂的流程图。它支持拖放、连接线、锚点等功能。
5. Pinia:Pinia 是一个简单、灵活和可扩展的状态管理库,可以帮助您管理应用程序的状态。
6. Sortable:Sortable 是一个 JavaScript 库,可以使您的列表可排序。它支持拖放、滑动等功能。
下面是一个简单的示例,演示如何使用这些技术和工具来构建一个完整的流程图:
1. 首先,您需要安装 Vite,可以使用以下命令:
```
npm install -g vite
```
2. 创建一个新的 Vue 3 项目,可以使用以下命令:
```
npm init vite-app my-project
```
3. 安装 Element Plus、Jsplumb、Pinia 和 Sortable,可以使用以下命令:
```
npm install element-plus jsplumb pinia sortable
```
4. 在 App.vue 文件中,添加一个包含 jsplumb 实例的 div 元素:
```vue
<template>
<div id="app">
<div id="jsplumb"></div>
</div>
</template>
```
5. 在 setup 方法中,创建一个 jsplumb 实例并将其挂载到 div 元素上:
```vue
<script>
import jsPlumb from 'jsplumb'
export default {
setup() {
const jsplumbInstance = new jsPlumb.jsPlumb()
jsplumbInstance.setContainer('jsplumb')
return {
jsplumbInstance,
}
},
}
</script>
```
6. 创建一个 Flowchart 组件,在其中添加一个包含 Sortable 列表的 div 元素:
```vue
<template>
<div class="flowchart">
<div class="flowchart-nodes" ref="nodes">
<div v-for="node in nodes" :key="node.id" class="flowchart-node">
{{ node.label }}
</div>
</div>
<div class="flowchart-connections" ref="connections"></div>
</div>
</template>
```
7. 在 setup 方法中,使用 Pinia 创建一个 store,用于管理节点和连接线的状态:
```vue
<script>
import { defineComponent } from 'vue'
import { createPinia } from 'pinia'
const store = createPinia()
export default defineComponent({
setup() {
store.state.nodes = []
store.state.connections = []
return {
store,
}
},
})
</script>
```
8. 在 mounted 方法中,使用 Sortable 初始化节点列表:
```vue
<script>
import { defineComponent, onMounted } from 'vue'
import Sortable from 'sortablejs'
// ...
export default defineComponent({
setup() {
// ...
onMounted(() => {
Sortable.create(nodes.value, {
group: 'nodes',
sort: true,
animation: 150,
onEnd: (evt) => {
store.state.nodes.splice(evt.newIndex, 0, store.state.nodes.splice(evt.oldIndex, 1)[0])
},
})
})
return {
// ...
}
},
})
</script>
```
9. 创建一个 Node 组件,在其中添加一个包含节点内容的 div 元素:
```vue
<template>
<div class="node">
<div class="node-content">
<slot />
</div>
</div>
</template>
```
10. 在 Flowchart 组件中,使用 Node 组件来渲染节点:
```vue
<template>
<div class="flowchart">
<div class="flowchart-nodes" ref="nodes">
<Node v-for="node in store.state.nodes" :key="node.id">
{{ node.label }}
</Node>
</div>
<div class="flowchart-connections" ref="connections"></div>
</div>
</template>
```
11. 创建一个 Connection 组件,在其中添加一个包含连接线的 div 元素:
```vue
<template>
<div class="connection">
<div class="connection-line" ref="line"></div>
</div>
</template>
```
12. 在 Flowchart 组件中,使用 Connection 组件来渲染连接线:
```vue
<template>
<div class="flowchart">
<div class="flowchart-nodes" ref="nodes">
<Node v-for="node in store.state.nodes" :key="node.id">
{{ node.label }}
</Node>
</div>
<div class="flowchart-connections" ref="connections">
<Connection v-for="connection in store.state.connections" :key="connection.id" />
</div>
</div>
</template>
```
13. 在 setup 方法中,使用 jsplumb 实例初始化连接线:
```vue
<script>
import { defineComponent } from 'vue'
import jsPlumb from 'jsplumb'
// ...
export default defineComponent({
setup() {
// ...
onMounted(() => {
jsplumbInstance.ready(() => {
store.watch(
() => store.state.connections,
() => {
store.state.connections.forEach((connection) => {
const sourceNode = jsplumbInstance.getEndpoints(connection.sourceNodeId)[0]
const targetNode = jsplumbInstance.getEndpoints(connection.targetNodeId)[0]
const jsplumbConnection = jsplumbInstance.connect({
source: sourceNode,
target: targetNode,
anchors: ['Right', 'Left'],
endpoint: 'Blank',
connector: ['Flowchart', { cornerRadius: 5 }],
paintStyle: {
stroke: '#5c5c5c',
strokeWidth: 2,
},
})
connection.id = jsplumbConnection.id
})
},
{ immediate: true }
)
})
})
return {
// ...
}
},
})
</script>
```
14. 在 Node 组件上,使用 jsplumb 实例初始化可拖动和可连接的锚点:
```vue
<script>
import { defineComponent, onMounted } from 'vue'
import jsPlumb from 'jsplumb'
export default defineComponent({
setup(props, { slots }) {
const endpoint = jsplumbInstance.addEndpoint(self.value, {
anchor: ['Right', 'Left'],
endpoint: 'Blank',
connector: ['Flowchart', { cornerRadius: 5 }],
paintStyle: {
stroke: '#5c5c5c',
strokeWidth: 2,
},
isSource: true,
isTarget: true,
})
onMounted(() => {
jsplumbInstance.draggable(self.value, {
grid: [10, 10],
})
})
return {
endpoint,
}
},
})
</script>
```
15. 在 Node 组件上,使用 jsplumb 实例监听锚点连接事件,并在连接时更新 store 中的连接线状态:
```vue
<script>
// ...
export default defineComponent({
setup(props, { slots }) {
// ...
endpoint.bind('connection', (connection) => {
const sourceNodeId = connection.sourceId.split('-')[1]
const targetNodeId = connection.targetId.split('-')[1]
store.state.connections.push({
id: null,
sourceNodeId,
targetNodeId,
})
})
return {
endpoint,
}
},
})
</script>
```
通过以上步骤,您可以使用 Vite、Element Plus、Jsplumb、Pinia 和 Sortable 来构建一个完整的流程图应用程序。
阅读全文
相关推荐
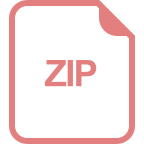
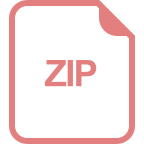
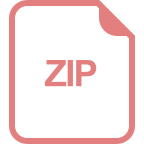
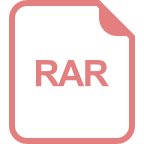
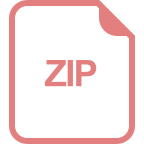
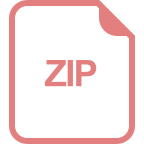



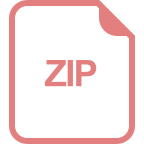
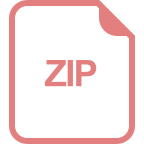
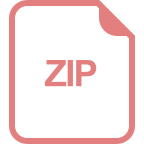
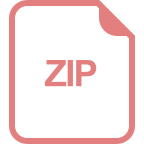
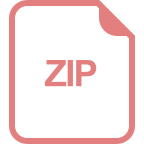
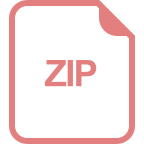
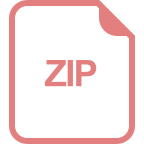
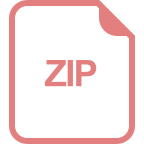
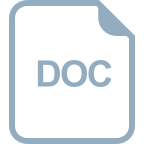
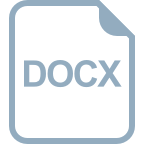