uniapp 读去 store 数据
时间: 2023-08-05 10:08:58 浏览: 108
在uniapp中,可以通过vuex来管理store数据。如果要读取store数据,可以使用以下方法:
1. 在组件中使用计算属性获取store数据:
```javascript
computed: {
myData() {
return this.$store.state.myData;
}
}
```
2. 在组件中使用$store.getters获取store数据:
```javascript
computed: {
myData() {
return this.$store.getters.myData;
}
}
```
其中,myData为store中保存的数据的名称。需要在store中定义对应的state或getter。
同时,需要在main.js中引入vuex并且将store注入到根组件中:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
import store from './store'
Vue.use(Vuex)
new Vue({
store,
...
})
```
相关问题
uniapp vue3 store
Uniapp 是一个跨平台的开发框架,可以使用 Vue.js 来开发多端应用。对于 Vue 3 的 store,Uniapp 也提供了相应的支持。
在 Uniapp 中,可以使用 Vuex 来管理应用的状态。在 Vue 3 中,Vuex 也有相应的更新来适配 Composition API。你可以按照以下步骤来创建和使用 Vuex store。
1. 首先,在项目的根目录下,创建一个 `store` 目录,用来存放 Vuex 的相关文件。
2. 在 `store` 目录内,创建一个 `index.js` 文件,用来初始化 Vuex 的 store。
3. 在 `index.js` 中,引入 Vue 和 Vuex:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
// 创建一个 store 实例
const store = new Vuex.Store({
state: {
// 在这里定义你的状态
},
mutations: {
// 在这里定义你的变更方法
},
actions: {
// 在这里定义你的异步操作方法
},
getters: {
// 在这里定义你的计算属性
}
})
export default store
```
4. 在你的应用主文件(一般是 `main.js` 或者 `App.vue`)中,引入并使用该 store:
```javascript
import store from './store'
new Vue({
store,
render: h => h(App)
}).$mount('#app')
```
现在,你就可以在应用的任何组件中使用该 store 来管理应用的状态了。你可以通过 `this.$store` 来访问 store 的属性和方法,例如:
```javascript
// 读取状态
const count = this.$store.state.count
// 提交变更
this.$store.commit('increment', payload)
// 分发异步操作
this.$store.dispatch('fetchData')
```
以上就是在 Uniapp 中使用 Vue 3 的 store(基于 Vuex)的基本步骤。你可以根据自己的需求,在 store 中定义状态、变更方法、异步操作方法和计算属性,来管理应用的数据和逻辑。
uniapp上架AppStore
uniapp上架AppStore需要以下步骤:
1. 兼容检查:在上架之前,确保你的uniapp项目在iOS平台上完全兼容,并且没有任何功能或兼容性问题。
2. 账号申请:在App Store上架应用程序之前,你需要拥有一个有效的苹果开发者账号。苹果开发者账号可以在苹果开发者官网上进行申请。
3. 证书申请:在准备上架之前,你需要生成一个用于签名和发布应用程序的证书。这个证书可以通过苹果开发者账号管理中心的证书部分进行申请和生成。
4. 发布准备:在提交应用程序之前,你需要准备应用程序的基本信息,包括应用名称、图标、描述、截图等。确保这些信息准确无误。
5. App Store上架:使用苹果开发者账号登录到App Store Connect,选择创建新App,填写必要的信息,上传应用程序包,完成应用程序的上架。
6. 审核后续:提交应用程序后,苹果审核团队将对应用程序进行审核。可能需要等待一段时间才能收到审核结果。如果应用程序通过审核,则会在App Store上架成功。
请注意,上架过程中可能会需要其他额外的步骤和信息,具体要根据你的应用程序和苹果开发者账号的要求来决定。建议参考苹果官方文档和开发者支持中心上的详细指南和教程,以确保顺利上架你的uniapp应用程序到App Store。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [利用uni-app 开发的iOS app 发布到App Store全流程](https://blog.csdn.net/y19910825/article/details/121497299)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
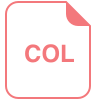
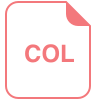
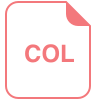
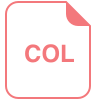
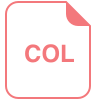







