mybatis-plus 外键关联
时间: 2023-09-21 12:09:52 浏览: 421
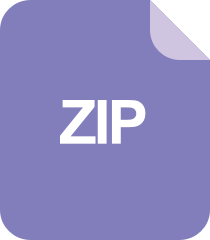
mybatis-plus
Mybatis-Plus 支持外键关联的操作,具体实现方式可以分为两种:
1. 通过 `@TableField` 注解指定关联属性,使用 `@TableField(exist = false)` 标识该属性为非数据库字段,然后通过 `@TableField(value = "foreign_key")` 指定关联的外键字段。具体代码如下:
```java
@Data
public class User {
private Long id;
private String name;
private Integer age;
@TableField(value = "dept_id")
private Long deptId;
}
@Data
public class Dept {
private Long id;
private String name;
}
@Mapper
public interface UserMapper extends BaseMapper<User> {
@Select("select u.*, d.name as dept_name from user u left join dept d on u.dept_id = d.id where u.id=#{id}")
User selectUserWithDept(Long id);
}
```
2. 使用 Mybatis-Plus 提供的 `@TableName` 注解的 `excludeProperty` 属性指定不需要插入的属性,然后使用 `@TableId` 注解指定主键,使用 `@TableField` 注解指定外键。具体代码如下:
```java
@Data
@TableName(excludeProperty = "dept")
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
@TableField("dept_id")
private Long deptId;
@TableField(exist = false)
private Dept dept;
}
@Data
@TableName("dept")
public class Dept {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
}
@Mapper
public interface UserMapper extends BaseMapper<User> {
@Select("select u.*, d.name as dept_name from user u left join dept d on u.dept_id = d.id where u.id=#{id}")
@Results({
@Result(column = "dept_id", property = "deptId"),
@Result(column = "dept_name", property = "dept.name")
})
User selectUserWithDept(Long id);
}
```
这里使用 `@Results` 注解来指定结果集中的属性映射关系,其中 `column` 指定查询结果中的列名,`property` 指定实体类中的属性名。
阅读全文
相关推荐



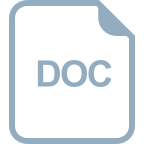
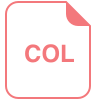











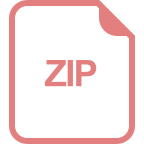