2)整型数据用顺序表作存储结构: ①读人一个整数序列 L(要求该整数序列从磁盘文件读取),生成一棵二叉排序树 T。 ②对二叉排序树 作中序遍历,输出结果。 ③ 计算二叉排序树 T 查找成功的平均查找长度,输出结果。 ④输人元素 ×,查找二叉排序树 T。若存在含x 的结点,则删除除该结点,并作中序遍历。否则输出没有该结点。用C语言写出代码
时间: 2024-03-17 18:42:58 浏览: 22
好的,下面是用 C 语言实现这道题目的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 插入节点
void insert(TreeNode** root, int val) {
if (*root == NULL) {
*root = (TreeNode*) malloc(sizeof(TreeNode));
(*root)->val = val;
(*root)->left = NULL;
(*root)->right = NULL;
return;
}
if ((*root)->val == val) {
return;
} else if ((*root)->val > val) {
insert(&((*root)->left), val);
} else {
insert(&((*root)->right), val);
}
}
// 中序遍历
void inorder(TreeNode* root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->val);
inorder(root->right);
}
// 计算平均查找长度
void calc_avg_length(TreeNode* root, int depth, int* count, int* sum) {
if (root == NULL) {
return;
}
(*count)++;
(*sum) += depth;
calc_avg_length(root->left, depth + 1, count, sum);
calc_avg_length(root->right, depth + 1, count, sum);
}
// 查找节点
TreeNode* find(TreeNode* root, int val) {
if (root == NULL) {
return NULL;
}
if (root->val == val) {
return root;
} else if (root->val > val) {
return find(root->left, val);
} else {
return find(root->right, val);
}
}
// 删除节点
void delete(TreeNode** root, int val) {
if (*root == NULL) {
return;
}
if ((*root)->val == val) {
if ((*root)->left == NULL && (*root)->right == NULL) {
free(*root);
*root = NULL;
} else if ((*root)->left == NULL) {
TreeNode* tmp = *root;
*root = (*root)->right;
free(tmp);
} else if ((*root)->right == NULL) {
TreeNode* tmp = *root;
*root = (*root)->left;
free(tmp);
} else {
TreeNode* tmp = (*root)->right;
while (tmp->left != NULL) {
tmp = tmp->left;
}
(*root)->val = tmp->val;
delete(&((*root)->right), tmp->val);
}
} else if ((*root)->val > val) {
delete(&((*root)->left), val);
} else {
delete(&((*root)->right), val);
}
}
int main() {
// 读取整数序列 L
FILE* fp = fopen("input.txt", "r");
if (fp == NULL) {
printf("Failed to open file!\n");
return 1;
}
int n;
TreeNode* root = NULL;
while (fscanf(fp, "%d", &n) != EOF) {
insert(&root, n);
}
fclose(fp);
// 对二叉排序树 T 作中序遍历
printf("Inorder traversal:\n");
inorder(root);
printf("\n");
// 计算二叉排序树 T 查找成功的平均查找长度
int count = 0, sum = 0;
calc_avg_length(root, 1, &count, &sum);
printf("Average search length: %.2f\n", (float) sum / count);
// 输入元素 x,查找二叉排序树 T
int x;
printf("Enter a number to search and delete: ");
scanf("%d", &x);
TreeNode* node = find(root, x);
if (node == NULL) {
printf("No such node!\n");
} else {
delete(&root, x);
printf("Inorder traversal after deletion:\n");
inorder(root);
printf("\n");
}
return 0;
}
```
在这段代码中,我们首先定义了二叉树节点的结构体,然后实现了二叉排序树的插入、中序遍历、计算平均查找长度、查找和删除操作。在主函数中,我们读取输入文件中的整数序列,并依次插入到二叉排序树中,然后对二叉排序树进行中序遍历和计算平均查找长度。最后,程序要求我们输入一个元素 x,查找并删除二叉排序树中的该元素,并输出删除后的中序遍历结果。
相关推荐
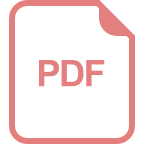
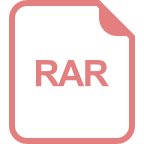
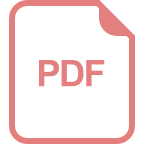
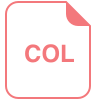
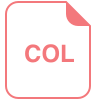
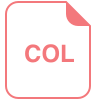
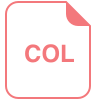
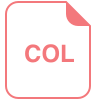










