package dailyioStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; public class FileOutputStreamDemo01 { public static void main(String[] args) throws IOException { FileOutputStream fos = new FileOutputStream("src\\dailyioStream\\fos.txt"); /* 这里创建了字节输出流对象 fos 创建字节输出流对象这里一共有三步操作: 1.调用了系统功能创建了文件 2.创建了字节流输出对象 3.让字节输出流对象指向创建好的文件 */ fos.write(97);//写到低层是97(ASCII码),但是我们打开文件内容是a fos.write(57); fos.write(55); fos.close();//释放资源,关闭此文件输出流释放并与此流相关联的任何系统资源 } }
时间: 2024-02-15 08:27:31 浏览: 60
这段代码是用Java语言实现的文件输出,将字符'a'、'9'和'7'写入到指定的文件夹和文件名中的fos.txt文件里。其中,先通过FileOutputStream类创建一个输出流对象fos,然后通过调用该对象的write()方法将字符写入到输出流中,最后通过close()方法关闭输出流并释放相关的系统资源。
相关问题
package test; import java.io.*; public class test { public static void main(String[] args) throws IOException { // TODO Auto-generated method stub FileOutputStream fis=new FileOutputStream("cat.jpg"); FileInputStream fos=new FileInputStream("cats.jpg"); BufferedOutputStream bis=new BufferedOutputStream(fis); BufferedInputStream bos=new BufferedInputStream(fos); } byte[] bytes=new byte[1024]; int len=-1; while((len=bis.read(bytes))!=-1) { bos.write(bytes,0,len); } } } 请帮我更改错误并进行说明
代码中存在一些语法错误和逻辑错误,下面是修改后的代码,并附有注释说明:
```java
package test;
import java.io.*;
public class Test {
public static void main(String[] args) throws IOException {
// 创建文件输出流并指定输出文件名为cat.jpg
FileOutputStream fos = new FileOutputStream("cat.jpg");
// 创建文件输入流并指定输入文件名为cats.jpg
FileInputStream fis = new FileInputStream("cats.jpg");
// 创建缓冲输出流并将文件输出流作为参数传入
BufferedOutputStream bos = new BufferedOutputStream(fos);
// 创建缓冲输入流并将文件输入流作为参数传入
BufferedInputStream bis = new BufferedInputStream(fis);
byte[] bytes = new byte[1024];
int len = -1;
// 读取缓冲输入流中的数据到字节数组中,并将读取的字节数赋值给len变量
while ((len = bis.read(bytes)) != -1) {
// 将字节数组中的数据写入到缓冲输出流中
bos.write(bytes, 0, len);
}
// 关闭缓冲输出流、文件输出流、缓冲输入流和文件输入流
bos.close();
fos.close();
bis.close();
fis.close();
}
}
```
注释说明:
1. 创建文件输出流和文件输入流时需要指定文件名(包括文件路径),否则会报FileNotFoundException异常。
2. 创建缓冲输出流和缓冲输入流时需要将文件输出流和文件输入流作为参数传入。
3. 在读取缓冲输入流中的数据时需要使用while循环,每次循环都会将读取的字节数赋值给len变量,当读取到文件末尾时,len的值为-1。
4. 在写入缓冲输出流时需要指定写入的字节数组、起始偏移量和写入长度。
5. 在程序结束时需要关闭所有的流,否则会导致文件资源无法释放,造成内存泄漏。
阅读全文
相关推荐
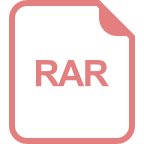
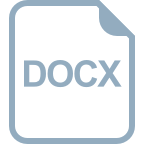
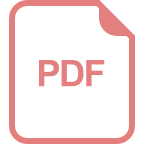
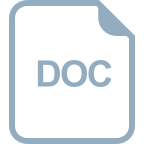
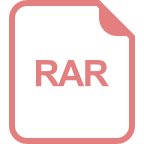
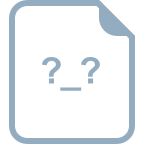
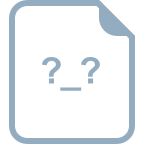
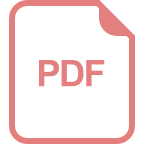
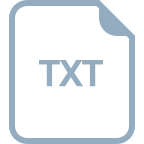
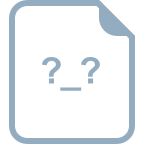
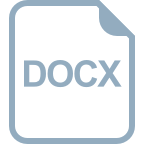
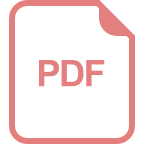
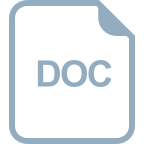
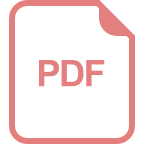
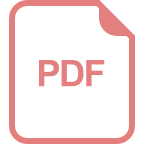
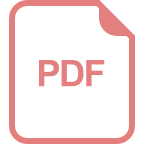
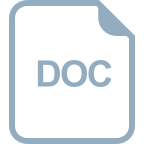
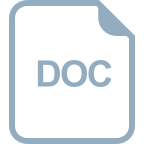