写一个三消游戏
时间: 2023-07-01 16:08:02 浏览: 214
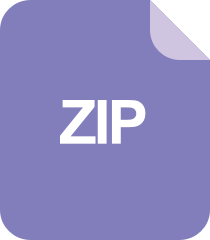
一个三消游戏demo

好的,我会给您写一个基础版的三消游戏。
首先,我们需要定义游戏的规则和逻辑。在三消游戏中,玩家需要通过交换两个相邻的方块来消除三个或三个以上的相同方块,每次消除可以得到一定的分数。当玩家无法再进行消除时,游戏结束。
接下来,我们需要设计游戏的界面和用户交互。游戏界面应该包括一个方块矩阵,并且在每个方块上显示不同的颜色或图案。玩家可以通过点击或滑动相邻的两个方块来进行交换。在每次交换后,程序需要判断是否有三个或三个以上的相同方块,如果有,则将它们从矩阵中移除,并且更新得分。当没有可消除的方块时,游戏结束。
下面是一个简单的代码示例:
```
import random
# 游戏设置
ROW = 8
COL = 8
BLOCK_SIZE = 50
MARGIN = 10
SCREEN_WIDTH = COL * BLOCK_SIZE + (COL + 1) * MARGIN
SCREEN_HEIGHT = ROW * BLOCK_SIZE + (ROW + 1) * MARGIN
BACKGROUND_COLOR = (200, 200, 200)
FONT_COLOR = (255, 255, 255)
# 游戏状态
SELECTED_BLOCK = None
SWAP_BLOCK = None
BLOCKS = []
SCORE = 0
# 初始化游戏窗口
pygame.init()
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("三消游戏")
font = pygame.font.SysFont(None, 36)
# 创建方块
def create_blocks():
global BLOCKS
BLOCKS = []
for r in range(ROW):
row = []
for c in range(COL):
color = random.choice([(255, 0, 0), (0, 255, 0), (0, 0, 255)])
block = pygame.Rect(
MARGIN + c * (BLOCK_SIZE + MARGIN),
MARGIN + r * (BLOCK_SIZE + MARGIN),
BLOCK_SIZE,
BLOCK_SIZE
)
row.append((block, color))
BLOCKS.append(row)
# 画方块
def draw_blocks():
for row in BLOCKS:
for block, color in row:
pygame.draw.rect(screen, color, block)
# 交换方块
def swap_blocks():
global BLOCKS, SELECTED_BLOCK, SWAP_BLOCK, SCORE
if SELECTED_BLOCK and SWAP_BLOCK:
r1, c1 = SELECTED_BLOCK
r2, c2 = SWAP_BLOCK
if abs(r1 - r2) + abs(c1 - c2) == 1:
BLOCKS[r1][c1], BLOCKS[r2][c2] = BLOCKS[r2][c2], BLOCKS[r1][c1]
if check_blocks():
SCORE += 10
else:
BLOCKS[r1][c1], BLOCKS[r2][c2] = BLOCKS[r2][c2], BLOCKS[r1][c1]
SELECTED_BLOCK = None
SWAP_BLOCK = None
# 检查方块是否可以消除
def check_blocks():
global BLOCKS
for r in range(ROW):
for c in range(COL):
if c + 2 < COL and BLOCKS[r][c][1] == BLOCKS[r][c+1][1] == BLOCKS[r][c+2][1]:
BLOCKS[r][c] = (BLOCKS[r][c][0], BACKGROUND_COLOR)
BLOCKS[r][c+1] = (BLOCKS[r][c+1][0], BACKGROUND_COLOR)
BLOCKS[r][c+2] = (BLOCKS[r][c+2][0], BACKGROUND_COLOR)
return True
elif r + 2 < ROW and BLOCKS[r][c][1] == BLOCKS[r+1][c][1] == BLOCKS[r+2][c][1]:
BLOCKS[r][c] = (BLOCKS[r][c][0], BACKGROUND_COLOR)
BLOCKS[r+1][c] = (BLOCKS[r+1][c][0], BACKGROUND_COLOR)
BLOCKS[r+2][c] = (BLOCKS[r+2][c][0], BACKGROUND_COLOR)
return True
return False
# 检查是否有可消除的方块
def check_gameover():
global BLOCKS
for r in range(ROW):
for c in range(COL):
if c + 2 < COL and BLOCKS[r][c][1] == BLOCKS[r][c+1][1] == BLOCKS[r][c+2][1]:
return False
elif r + 2 < ROW and BLOCKS[r][c][1] == BLOCKS[r+1][c][1] == BLOCKS[r+2][c][1]:
return False
return True
# 显示得分
def show_score():
text = f"得分: {SCORE}"
score_text = font.render(text, True, FONT_COLOR)
screen.blit(score_text, (MARGIN, SCREEN_HEIGHT - MARGIN - 50))
# 游戏主循环
def main_loop():
global SELECTED_BLOCK, SWAP_BLOCK
create_blocks()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
c = (x - MARGIN) // (BLOCK_SIZE + MARGIN)
r = (y - MARGIN) // (BLOCK_SIZE + MARGIN)
if r < ROW and c < COL:
if not SELECTED_BLOCK:
SELECTED_BLOCK = (r, c)
elif not SWAP_BLOCK:
SWAP_BLOCK = (r, c)
swap_blocks()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
running = False
screen.fill(BACKGROUND_COLOR)
draw_blocks()
show_score()
pygame.display.update()
if check_gameover():
create_blocks()
SCORE = 0
SELECTED_BLOCK = None
SWAP_BLOCK = None
pygame.quit()
if __name__ == "__main__":
main_loop()
```
这段代码实现了一个简单的三消游戏,您可以根据自己的需求进行修改和优化。
阅读全文
相关推荐






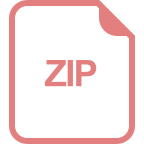
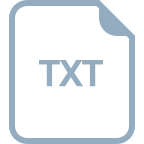
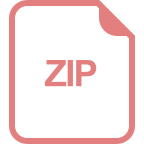
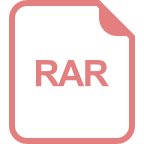
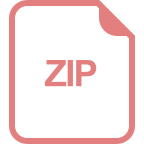
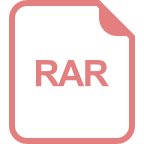
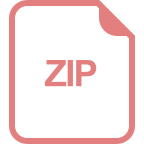
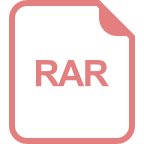
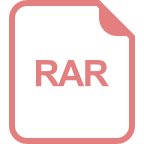
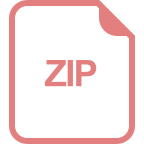