通过StreamReader怎么获取文件第一行和最后一行并打印出来
时间: 2024-01-22 16:02:13 浏览: 135
可以通过以下代码来实现:
```csharp
using System.IO;
// 读取文件的第一行和最后一行
string firstLine, lastLine;
using (StreamReader reader = new StreamReader("filename.txt"))
{
firstLine = reader.ReadLine(); // 读取第一行
string line;
while ((line = reader.ReadLine()) != null) // 读取最后一行
{
lastLine = line;
}
}
// 打印第一行和最后一行
Console.WriteLine("第一行:" + firstLine);
Console.WriteLine("最后一行:" + lastLine);
```
这段代码首先使用 `StreamReader` 打开文件,然后通过 `ReadLine()` 方法读取第一行。接着,使用一个 `while` 循环读取文件的每一行,直到读到最后一行,将最后一行的内容赋值给 `lastLine` 变量。最后,使用 `Console.WriteLine()` 打印出第一行和最后一行的内容。
相关问题
C#读取txt文件某一行并打印
在C#中,你可以使用`StreamReader`类来逐行读取txt文件。以下是一个简单的示例,演示如何读取并打印指定行的内容:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
// 文件路径
string filePath = "path_to_your_file.txt";
try
{
// 创建StreamReader实例,并打开文件
using (StreamReader reader = new StreamReader(filePath))
{
// 如果你想读取第n行,可以先获取总行数,然后减去1得到索引
int lineNumber = 5; // 假设你想读第5行
int lineCount = CountLines(reader); // 获取总行数
if (lineNumber <= lineCount)
{
// 读取指定行
string line = reader.ReadLine(lineNumber - 1);
Console.WriteLine("第{0}行内容: {1}", lineNumber, line);
}
else
{
Console.WriteLine("指定的行号超出了文件的实际行数。");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"发生错误: {ex.Message}");
}
}
// 辅助函数,用于计算文件总行数
static int CountLines(StreamReader reader)
{
long currentLine = 0;
string line;
while ((line = reader.ReadLine()) != null)
{
currentLine++;
}
return (int)currentLine;
}
}
```
在这个例子中,你需要将`path_to_your_file.txt`替换为你要读取的txt文件的实际路径。如果你想打印当前行,只需省略行号部分。
c#读取txt文件最后一行
在 C# 中,可以使用 `StreamReader` 类以及其 `ReadLine` 或者 `ReadToEnd` 方法来读取 `.txt` 文件的最后一行。以下是两种方法:
1. **逐行读取并查找最后一行**:
```csharp
using System;
using System.IO;
string filePath = "path_to_your_file.txt"; // 替换为实际文件路径
// 打开文件
using (StreamReader reader = new StreamReader(filePath))
{
string line;
while ((line = reader.ReadLine()) != null) // 当达到文件尾部,readLine() 返回null
{
if (line.Trim() != "") // 如果不是空白行,那就是最后一行
lastLine = line; // 把非空行赋值给 lastLine 变量
}
}
// 现在 lastLine 存储了最后一行的内容
```
2. **一次性读取所有内容然后获取最后一行**:
```csharp
using System;
using System.IO;
string filePath = "path_to_your_file.txt";
// 一次性读取所有文本到字符串
string allText = File.ReadAllText(filePath);
// 获取最后一行
int index = allText.LastIndexOf("\n"); // 找到最后一个换行符的位置
if (index != -1)
{
lastLine = allText.Substring(index + 1); // 提取从换行符之后的部分作为最后一行
}
else // 如果没有换行符(即整个文件只有一行)
{
lastLine = allText;
}
// lastLine 现在存储了最后一行的内容
```
请注意将 `filePath` 替换为你需要读取的实际文件路径。
相关推荐
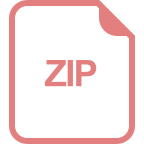
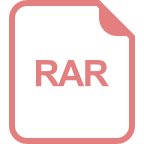












