利用数据结构与算法完成简单计算器的c语言代码
时间: 2023-08-05 07:40:03 浏览: 108
好的,我可以给你提供一个基于栈的简单计算器的C语言代码。代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_SIZE 100
typedef struct {
int top;
int data[MAX_SIZE];
} Stack;
void stack_push(Stack *stack, int value) {
if (stack->top == MAX_SIZE - 1) {
printf("Error: stack overflow\n");
exit(-1);
}
stack->data[++stack->top] = value;
}
int stack_pop(Stack *stack) {
if (stack->top == -1) {
printf("Error: stack underflow\n");
exit(-1);
}
return stack->data[stack->top--];
}
int stack_top(Stack *stack) {
if (stack->top == -1) {
printf("Error: stack underflow\n");
exit(-1);
}
return stack->data[stack->top];
}
int stack_empty(Stack *stack) {
return stack->top == -1;
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int operator_priority(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
int calculate(int a, int b, char operator) {
switch (operator) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return 0;
}
}
int evaluate_expression(char *expression) {
Stack num_stack;
Stack op_stack;
num_stack.top = -1;
op_stack.top = -1;
while (*expression != '\0') {
if (isdigit(*expression)) {
int num = 0;
while (isdigit(*expression)) {
num = num * 10 + (*expression - '0');
expression++;
}
stack_push(&num_stack, num);
} else if (is_operator(*expression)) {
while (!stack_empty(&op_stack) && operator_priority(*expression) <= operator_priority(stack_top(&op_stack))) {
int b = stack_pop(&num_stack);
int a = stack_pop(&num_stack);
char op = stack_pop(&op_stack);
int result = calculate(a, b, op);
stack_push(&num_stack, result);
}
stack_push(&op_stack, *expression);
expression++;
} else {
expression++;
}
}
while (!stack_empty(&op_stack)) {
int b = stack_pop(&num_stack);
int a = stack_pop(&num_stack);
char op = stack_pop(&op_stack);
int result = calculate(a, b, op);
stack_push(&num_stack, result);
}
return stack_pop(&num_stack);
}
int main() {
char expression[MAX_SIZE];
printf("Enter an expression: ");
fgets(expression, MAX_SIZE, stdin);
int result = evaluate_expression(expression);
printf("Result: %d\n", result);
return 0;
}
```
这个代码实现了基于栈的简单计算器,包括栈的实现,操作符优先级的比较,后缀表达式的计算等。你可以将这段代码复制到你的编译器中编译运行,然后输入一个表达式进行计算。如果你有任何疑问,可以随时问我。
阅读全文
相关推荐








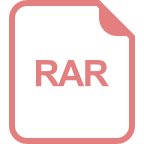




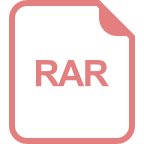
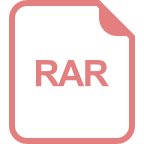

