ActionFilterAttribute解密URL
时间: 2023-07-14 11:56:59 浏览: 95
在ASP.NET MVC中,可以通过ActionFilterAttribute类来实现解密URL。具体步骤如下:
1. 创建一个ActionFilterAttribute类,用于对URL进行解密。
```
public class DecryptUrlAttribute : ActionFilterAttribute
{
private string _key;
public DecryptUrlAttribute(string key)
{
_key = key;
}
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
var request = filterContext.HttpContext.Request;
// 获取加密后的URL
var encryptedUrl = request.QueryString["url"];
// 如果加密后的URL不存在,则继续执行下一个Action Filter
if (string.IsNullOrEmpty(encryptedUrl))
{
base.OnActionExecuting(filterContext);
return;
}
// 解密URL
var decryptedUrl = Decrypt(encryptedUrl, _key);
// 将解密后的URL设置为Action参数
filterContext.ActionParameters["url"] = decryptedUrl;
base.OnActionExecuting(filterContext);
}
private string Decrypt(string encryptedText, string key)
{
byte[] encryptedBytes = Convert.FromBase64String(encryptedText);
byte[] keyBytes = Encoding.UTF8.GetBytes(key);
SymmetricAlgorithm algorithm = Aes.Create();
algorithm.Key = keyBytes;
MemoryStream memoryStream = new MemoryStream(encryptedBytes);
CryptoStream cryptoStream = new CryptoStream(memoryStream,
algorithm.CreateDecryptor(),
CryptoStreamMode.Read);
byte[] plainBytes = new byte[encryptedBytes.Length];
int decryptedByteCount = cryptoStream.Read(plainBytes, 0, plainBytes.Length);
return Encoding.UTF8.GetString(plainBytes, 0, decryptedByteCount);
}
}
```
在此示例中,DecryptUrlAttribute类继承自ActionFilterAttribute类,并在OnActionExecuting方法中对URL进行解密。在解密过程中,使用了之前提到的加密和解密方法。
2. 在Controller中使用DecryptUrlAttribute类。
在需要解密URL的Controller方法上加上[DecryptUrl]特性即可。例如:
```
[DecryptUrl("mykey")]
public ActionResult MyAction(string url)
{
// 处理解密后的URL
// ...
}
```
在此示例中,[DecryptUrl("mykey")]表示使用密钥"mykey"来解密URL。在MyAction方法中,参数url会被自动赋值为解密后的URL。
需要注意的是,使用此方法解密URL时,需要将加密后的URL作为QueryString的一部分传递。例如:
```
http://example.com/MyController/MyAction?url=encryptedUrl
```
其中,encryptedUrl为加密后的URL。
阅读全文
相关推荐
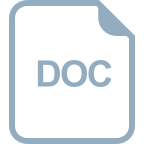
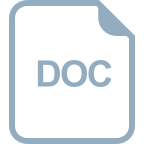
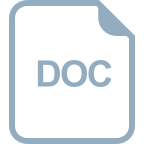
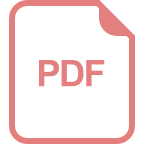
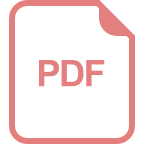
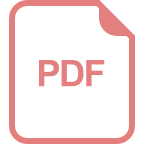
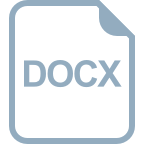
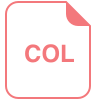




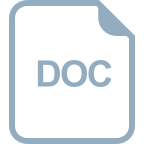
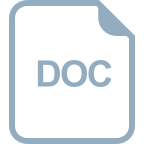
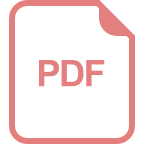
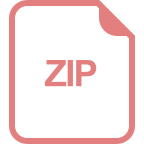
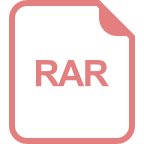
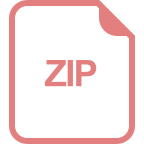
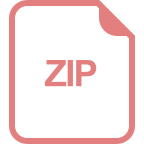