程序员,请创建一个Java游戏项目名叫www,包名叫www,并在包内创建一个login类,login类实现一个带有登录注册并连接数据库的游戏窗口,以代码形式并附上中文注释,然后建个main类来实现主页面,主页面有三个按钮,分别是‘开始游戏’,‘选择英雄机’,‘设置’,登陆成功后就跳转到主页面。请以代码形式呈现
时间: 2024-03-10 21:51:03 浏览: 20
好的,以下是Java代码:
首先创建Java项目`www`,并在包`www`中创建`Login`类:
```java
package www;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
public class Login extends JFrame implements ActionListener {
private JTextField tfUserName;
private JPasswordField tfPassword;
private JButton btnLogin, btnRegister;
private static final String DB_URL = "jdbc:mysql://localhost:3306/game";
private static final String USER = "root";
private static final String PASS = "password";
public Login() {
initUI();
}
private void initUI() {
setTitle("游戏登录");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new BorderLayout());
JPanel panelNorth = new JPanel(new FlowLayout(FlowLayout.LEFT));
JPanel panelCenter = new JPanel(new FlowLayout(FlowLayout.CENTER));
JPanel panelSouth = new JPanel(new FlowLayout(FlowLayout.CENTER));
JLabel lblUserName = new JLabel("用户名:");
tfUserName = new JTextField(20);
JLabel lblPassword = new JLabel("密码:");
tfPassword = new JPasswordField(20);
btnLogin = new JButton("登录");
btnLogin.addActionListener(this);
btnRegister = new JButton("注册");
btnRegister.addActionListener(this);
panelNorth.add(lblUserName);
panelNorth.add(tfUserName);
panelNorth.add(lblPassword);
panelNorth.add(tfPassword);
panelCenter.add(btnLogin);
panelCenter.add(btnRegister);
panel.add(panelNorth, BorderLayout.NORTH);
panel.add(panelCenter, BorderLayout.CENTER);
panel.add(panelSouth, BorderLayout.SOUTH);
setContentPane(panel);
pack();
setLocationRelativeTo(null);
}
private boolean isValidUser(String userName, String password) {
boolean isValid = false;
try (Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
Statement stmt = conn.createStatement();) {
String sql = "SELECT * FROM user WHERE username='" + userName + "' AND password='" + password + "'";
ResultSet rs = stmt.executeQuery(sql);
if (rs.next()) {
isValid = true;
}
} catch (SQLException ex) {
System.err.println(ex);
}
return isValid;
}
private void registerUser(String userName, String password) {
try (Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
Statement stmt = conn.createStatement();) {
String sql = "INSERT INTO user (username, password) VALUES ('" + userName + "', '" + password + "')";
stmt.executeUpdate(sql);
} catch (SQLException ex) {
System.err.println(ex);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnLogin) {
String userName = tfUserName.getText();
String password = String.valueOf(tfPassword.getPassword());
if (isValidUser(userName, password)) {
JOptionPane.showMessageDialog(this, "登录成功!");
openMainPage();
} else {
JOptionPane.showMessageDialog(this, "用户名或密码错误!");
}
} else if (e.getSource() == btnRegister) {
String userName = tfUserName.getText();
String password = String.valueOf(tfPassword.getPassword());
registerUser(userName, password);
JOptionPane.showMessageDialog(this, "注册成功!");
}
}
private void openMainPage() {
Main main = new Main();
main.setVisible(true);
dispose();
}
}
```
在`Login`类中,我们实现了一个带有登录注册并连接数据库的游戏窗口。使用`JFrame`类来创建窗口界面,包括用户名输入框、密码输入框、登录按钮和注册按钮。通过`isValidUser`方法来验证用户是否合法,并通过`registerUser`方法来注册新用户。
接下来是`Main`类,用于实现主页面,包含三个按钮:开始游戏、选择英雄机和设置:
```java
package www;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Main extends JFrame {
public Main() {
initUI();
}
private void initUI() {
setTitle("游戏主页面");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new BorderLayout());
JPanel panelCenter = new JPanel(new FlowLayout(FlowLayout.CENTER));
JButton btnStartGame = new JButton("开始游戏");
JButton btnSelectHero = new JButton("选择英雄机");
JButton btnSetting = new JButton("设置");
panelCenter.add(btnStartGame);
panelCenter.add(btnSelectHero);
panelCenter.add(btnSetting);
panel.add(panelCenter, BorderLayout.CENTER);
setContentPane(panel);
pack();
setLocationRelativeTo(null);
}
}
```
在`Main`类中,我们创建了一个`JFrame`窗口,并添加了三个按钮:开始游戏、选择英雄机和设置。最后,我们将`panelCenter`添加到`panel`中,并将其设置为窗口的内容面板。在`initUI`方法中,使用了`BorderLayout`和`FlowLayout`布局管理器来实现按钮的布局。
最后,在`Login`类中,在`isValidUser`方法中添加以下代码,以实现在登录成功后打开主页面:
```java
private void openMainPage() {
Main main = new Main();
main.setVisible(true);
dispose();
}
```
在登录成功后,通过`openMainPage`方法打开`Main`类,并关闭当前的登录窗口。
相关推荐
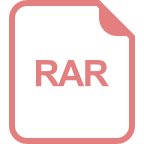
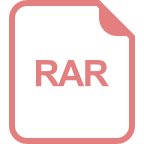
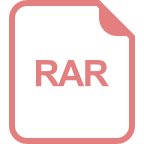














