使用 _thread 模块如何创建多线程,举个例子python
时间: 2023-07-15 16:15:28 浏览: 58
好的,您的问题是关于使用_thread模块创建多线程的例子。以下是一个使用_thread模块创建两个线程的例子:
```
import _thread
import time
# 定义一个函数,用于打印当前线程名字和时间
def print_time(threadName, delay):
count = 0
while count < 5:
time.sleep(delay)
count += 1
print("%s: %s" % (threadName, time.ctime(time.time())))
try:
# 创建线程1
_thread.start_new_thread( print_time, ("Thread-1", 2, ) )
# 创建线程2
_thread.start_new_thread( print_time, ("Thread-2", 4, ) )
except:
print("Error: 无法启动线程")
# 让主线程等待子线程结束
while 1:
pass
```
在上面的例子中,我们定义了一个名为print_time的函数,这个函数会打印当前线程的名字和当前时间,然后休眠一段时间。接下来,在try语句块中,我们使用_thread.start_new_thread()函数分别创建了两个线程,这两个线程都调用了print_time函数,并传入不同的线程名字和休眠时间。最后,我们让主线程等待子线程结束,以保证程序不会过早退出。
相关问题
使用_thread模块创建多线程的例子python
好的,以下是使用_thread模块创建多线程的python代码示例:
```
import _thread
# 定义线程函数
def worker(threadID, delay):
while True:
print("Thread ID {} is running".format(threadID))
time.sleep(delay)
# 创建线程
try:
_thread.start_new_thread(worker, (1, 1))
_thread.start_new_thread(worker, (2, 2))
_thread.start_new_thread(worker, (3, 3))
except:
print("Error: unable to start thread")
while True:
pass # 主线程无限运行
```
在这个例子中,我们定义了一个worker函数,它会在不断循环的过程中打印当前线程的ID,并且使用time模块中的sleep函数,让线程在打印之间暂停一段时间。接着我们使用_thread模块的start_new_thread函数来创建三个线程,并分别给它们传递不同的线程ID和延迟时间。最后,我们让主线程无限运行,以保证程序不会退出。
如何使用Python的内置模块threading来创建多个线程
可以使用Python的内置模块threading来创建多个线程,具体步骤如下:
1.导入threading模块:`import threading`
2.定义一个函数,作为线程的执行体。
3.创建多个线程:`t = threading.Thread(target=func, args=(arg1, arg2, ...))`,其中func表示要执行的函数,args表示传递给函数的参数。
4.启动线程:`t.start()`。
下面是一个简单的示例代码,创建两个线程并行执行:
```python
import threading
def func(name):
print('Hello, %s!' % name)
t1 = threading.Thread(target=func, args=('Alice',))
t2 = threading.Thread(target=func, args=('Bob',))
t1.start()
t2.start()
t1.join()
t2.join()
print('All threads have finished.')
```
在这个例子中,定义了一个函数func,用于输出一句问候语。使用threading.Thread创建两个线程t1和t2,分别传递了不同的参数。然后使用t1.start()和t2.start()启动线程,使用t1.join()和t2.join()等待线程执行完毕,最后输出一句提示语,表示所有线程都已经执行完毕。
相关推荐
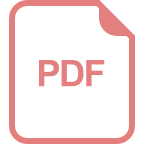
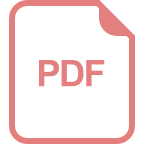
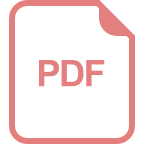












