用c++定义一个基类有姓名、性别、年龄,再由基类派生出教师类和学生类。教师类增加工号、职称和工资,学生类增加学号、班级、专业和入学成绩。 编程要求: 1、 设计虚函数输出教师和学生的基本信息; 2、 计算教师的平均工资并输出; 3、 使用友元函数重载运算符“<”,找出入学成绩最高的学生并输出;
时间: 2024-01-27 18:05:15 浏览: 49
好的,下面是C++代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name; //姓名
string gender; //性别
int age; //年龄
public:
Person(string n, string g, int a) : name(n), gender(g), age(a) {}
virtual void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
}
virtual ~Person() {}
};
class Teacher : public Person {
private:
int id; //工号
string title; //职称
double salary; //工资
public:
Teacher(string n, string g, int a, int i, string t, double s) : Person(n, g, a), id(i), title(t), salary(s) {}
void display() {
Person::display();
cout << "工号:" << id << endl;
cout << "职称:" << title << endl;
cout << "工资:" << salary << endl;
}
double getSalary() const { return salary; }
~Teacher() {}
};
class Student : public Person {
private:
int sid; //学号
string className; //班级
string major; //专业
double score; //入学成绩
public:
Student(string n, string g, int a, int id, string c, string m, double s) : Person(n, g, a), sid(id), className(c), major(m), score(s) {}
void display() {
Person::display();
cout << "学号:" << sid << endl;
cout << "班级:" << className << endl;
cout << "专业:" << major << endl;
cout << "入学成绩:" << score << endl;
}
friend bool operator<(const Student& s1, const Student& s2);
~Student() {}
};
bool operator<(const Student& s1, const Student& s2) {
return s1.score < s2.score;
}
double avgSalary(Teacher* t[], int n) {
double sum = 0;
for (int i = 0; i < n; i++) {
sum += t[i]->getSalary();
}
return sum / n;
}
int main() {
Teacher t("张三", "男", 35, 1001, "教授", 8000.0);
t.display();
cout << endl;
Student s1("李四", "男", 18, 2019001, "计算机科学与技术", "软件工程", 88.5);
Student s2("韩梅梅", "女", 19, 2019002, "计算机科学与技术", "人工智能", 92.0);
Student s3("王小明", "男", 20, 2019003, "计算机科学与技术", "网络安全", 85.0);
if (s1 < s2) {
s2.display();
}
else {
s1.display();
}
if (s3 < s2) {
s2.display();
}
else {
s3.display();
}
cout << endl;
Teacher* tArr[3];
tArr[0] = &t;
tArr[1] = new Teacher("李老师", "女", 28, 1002, "讲师", 5000.0);
tArr[2] = new Teacher("赵老师", "男", 40, 1003, "副教授", 6000.0);
cout << "教师平均工资:" << avgSalary(tArr, 3) << endl;
for (int i = 0; i < 3; i++) {
delete tArr[i];
}
return 0;
}
```
解释一下:
首先定义一个Person基类,包含姓名、性别和年龄属性,并且声明一个虚函数display,在派生类中重载这个函数。然后,分别定义教师类和学生类,分别增加了教师和学生的特有属性,并且重载了基类中的display函数。在学生类中,还定义了一个友元函数,用来比较学生的入学成绩大小。最后,定义一个计算教师平均工资的函数avgSalary,遍历教师数组并计算总工资,最后返回平均工资。在main函数中,创建一个教师对象和三个学生对象,并且调用它们的display函数输出信息。然后,使用重载运算符“<”找出入学成绩最高的学生并输出。最后,创建一个教师指针数组,并且遍历这个数组计算教师平均工资,并且释放动态分配的内存。
相关推荐







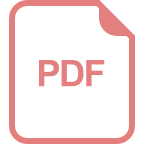
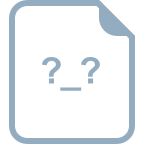
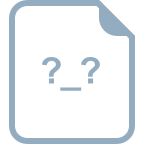
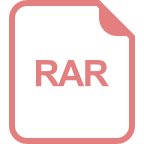