Stringstream
时间: 2023-10-23 20:12:15 浏览: 73
Stringstream is a class in C++ that allows you to read and write strings as if they were input/output streams. It is used for converting strings to numerical values, parsing strings, and formatting output. The class is defined in the header file <sstream>. The stringstream class allows you to treat a string as if it were a stream, which means you can use all the standard stream input/output operations on it. This includes inserting data into the string, extracting data from the string, and formatting the string using manipulators. The stringstream class is especially useful when you need to parse a string into individual tokens, or when you need to convert a string into a numerical value.
相关问题
stringstream
stringstream是C++中的一个类,用于将字符串与流进行互相转换。它可以将一个字符串转换为流,从而方便地从中读取数据;也可以将数据写入到流中,从而方便地将数据转换为字符串。stringstream类继承自istream和ostream类,因此可以使用输入和输出运算符来读取和写入数据。可以使用头文件<sstream>来包含stringstream类。
StringStream
C++中的stringstream是一个流类,它可以将字符串转换为数字、将数字转换为字符串等。stringstream类定义在头文件<sstream>中,使用时需要包含该头文件。
stringstream类的主要用法如下:
1. 将字符串转换为数字
可以使用stringstream类将字符串转换为数字,例如:
```
#include <iostream>
#include <sstream>
using namespace std;
int main()
{
string str = "123";
int num;
stringstream ss(str);
ss >> num;
cout << num << endl;
return ;
}
```
输出结果为:
```
123
```
2. 将数字转换为字符串
可以使用stringstream类将数字转换为字符串,例如:
```
#include <iostream>
#include <sstream>
using namespace std;
int main()
{
int num = 123;
stringstream ss;
ss << num;
string str = ss.str();
cout << str << endl;
return ;
}
```
输出结果为:
```
123
```
3. 将多个数据类型转换为字符串
可以使用stringstream类将多个数据类型转换为字符串,例如:
```
#include <iostream>
#include <sstream>
using namespace std;
int main()
{
int num = 123;
double d = 3.14;
string str = "hello";
stringstream ss;
ss << num << " " << d << " " << str;
string result = ss.str();
cout << result << endl;
return ;
}
```
输出结果为:
```
123 3.14 hello
```
以上就是stringstream类的主要用法。
阅读全文
相关推荐
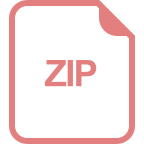
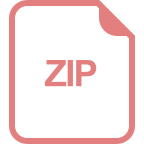
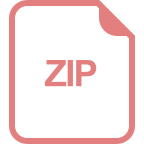
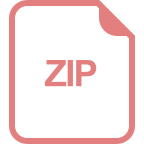
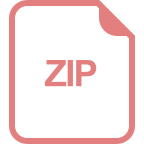
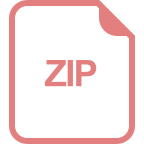
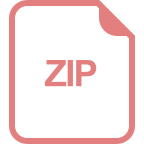
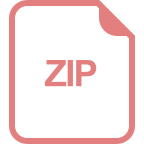
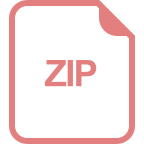
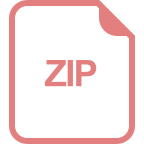
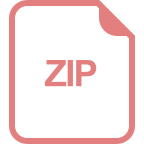
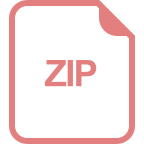
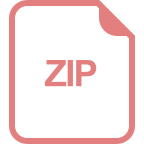
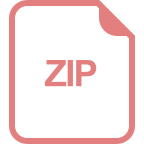