if __name__ == '__main__': ctx = app.app_context() ctx.push() db.drop_all() db.create_all() bk1 = Book(Sname='《刘小强虚构》', price=60,position:absoulte) bk2 = Book(Sname='《西游记》', price=40) bk3 = Book(Sname='《水浒传》', price=20) bk4 = Book(Sname='《三国演义》', price=30) bk5 = Book(Sname='《红楼梦》', price=40) # 把数据提交给用户会话 db.session.add_all([bk1, bk2, bk3, bk4, bk5]) # 提交会话 db.session.commit() ctx.pop() app.run(debug=True)
时间: 2024-03-26 21:36:52 浏览: 9
这段代码是一个 Python 程序的主程序入口,它首先创建了一个 Flask 应用程序上下文,然后使用该上下文对象来创建并初始化了一个新的数据库,接着向该数据库添加了5本书,并提交了该会话,最后运行了 Flask 应用程序。其中,`__name__` 是 Python 中一个特殊的变量,表示当前模块的名称,在这里用来检查是否是当前模块正在执行,以确保不会在其他模块引入该模块时执行该程序(这是一种常见的编程模式)。`app_context()` 方法是 Flask 应用程序对象的一个方法,用于创建一个应用程序上下文,`push()` 方法将该上下文推入上下文栈中,`drop_all()` 和 `create_all()` 方法是 SQLAlchemy 提供的 API,用于删除和创建数据库中所有的表。`bk1` 到 `bk5` 是 `Book` 类的实例对象,表示5本书,每本书包括名称和价格两个属性,其中 `bk1` 还有一个名为 `position` 的属性,值为 `absolute`。`db.session` 是 SQLAlchemy 中的一个会话对象,使用 `add_all()` 方法向该会话中添加了所有5本书,最后使用 `commit()` 方法提交该会话。`pop()` 方法将应用程序上下文对象从上下文栈中弹出,`run()` 方法运行 Flask 应用程序。
相关问题
ctx = mp.get_context("spawn")
这是使用 Python 的 multiprocessing 模块中的 get_context 方法创建进程上下文的代码。其中参数 "spawn" 表示使用 fork 系统调用创建新进程。在 Windows 平台上,由于没有 fork 系统调用,因此需要使用 "spawn" 或 "forkserver" 上下文来创建新的进程。这种方式可以在多进程编程中创建新的进程,以实现并行计算等任务。
from flask.globals import app_ctx
As an AI language model, I don't have access to the specific Flask application that you're working on. However, I can explain what the `app_ctx` object is in Flask.
The `app_ctx` object is a context object that is used to store application-level information in Flask. It is a global object that is created when the Flask application is initialized and it is destroyed when the application is shut down. The `app_ctx` object is used to store information that is shared across multiple requests, such as database connections, configuration settings, and other resources that are needed by the application.
To access the `app_ctx` object in Flask, you can use the `current_app.app_context()` method. This method returns a context object that provides access to the `app_ctx` object. Once you have the `app_ctx` object, you can access any application-level information that has been stored in it.
For example, to access a database connection that has been stored in the `app_ctx` object, you could use code like this:
```
from flask import current_app
with current_app.app_context():
db_conn = current_app.config['DB_CONN']
# Use the database connection here
```
In this code, the `current_app.config['DB_CONN']` line retrieves the database connection from the `app_ctx` object, which has been stored there as part of the application's configuration settings. The `with current_app.app_context():` line ensures that the `app_ctx` object is available for use within the code block.
相关推荐
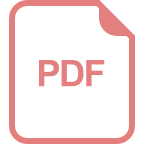













