Write pytorch-based Python code to implement a neural network that solves binary classification problem with an output layer of a positively weighted sub-network plus a negatively weighted sub-network,where the negative weight is random and take the opposite of the absolute value of the standard normal distribution, the exact value of the two weights are obtained from the objective function by Back propagation. The activation function is set to tanh.
时间: 2024-04-08 18:29:27 浏览: 63
Sure, here's an example of PyTorch-based code that implements a neural network with a positively weighted sub-network and a negatively weighted sub-network:
```python
import torch
import torch.nn as nn
import torch.optim as optim
# Define the neural network architecture
class BinaryClassifier(nn.Module):
def __init__(self):
super(BinaryClassifier, self).__init__()
self.positive_weight = nn.Parameter(torch.Tensor(1))
self.negative_weight = nn.Parameter(torch.Tensor(1))
self.negative_weight.data = -torch.abs(torch.randn(1))
self.fc1 = nn.Linear(in_features=2, out_features=10)
self.fc2 = nn.Linear(in_features=10, out_features=1)
self.activation = nn.Tanh()
def forward(self, x):
x = self.activation(self.fc1(x))
positive_output = self.fc2(x) * self.positive_weight
negative_output = self.fc2(x) * self.negative_weight
output = positive_output + negative_output
return output
# Create an instance of the neural network
model = BinaryClassifier()
# Define the loss function and optimizer
criterion = nn.BCEWithLogitsLoss()
optimizer = optim.SGD(model.parameters(), lr=0.1)
# Generate random training data
X = torch.randn(100, 2)
y = torch.randint(0, 2, (100, 1)).float()
# Training loop
for epoch in range(100):
optimizer.zero_grad()
output = model(X)
loss = criterion(output, y)
loss.backward()
optimizer.step()
if (epoch + 1) % 10 == 0:
print(f"Epoch {epoch+1}: Loss = {loss.item()}")
# Use the trained model for prediction
test_input = torch.Tensor([[0.5, 0.5]])
prediction = torch.sigmoid(model(test_input))
print(f"Prediction: {prediction.item()}")
```
In this code, we define a `BinaryClassifier` class that inherits from `nn.Module`. It contains the positive weight and negative weight as trainable parameters, which are initialized with random values. The architecture consists of two fully connected layers with a tanh activation function. The positive and negative outputs are computed by multiplying the output of the second fully connected layer with their respective weights. The final output is the sum of the positive and negative outputs.
We use the binary cross-entropy loss (`nn.BCEWithLogitsLoss`) as the loss function and stochastic gradient descent (`optim.SGD`) as the optimizer. Random training data is generated, and the model is trained for 100 epochs. The loss is printed every 10 epochs. Finally, the trained model is used to make a prediction on a test input.
Please note that the code above assumes you have PyTorch installed and imported appropriately.
阅读全文
相关推荐
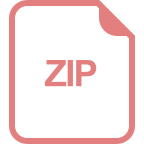
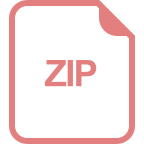
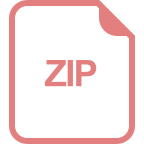













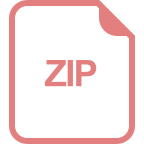
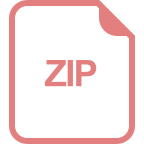