Design a simple Book Seller System with strategy pattern. (25 points) (a)You don’t care all the functions of the system. (b)There are three kinds of client which include VIPMember(0.9*price), NormalMember(1*price), SuperMember(0.8*price). They have the different discount when they buy book from the system. (c)You must use strategy pattern when you design the system. (d)Firstly, you should give the UML class diagram with the strategy pattern. (5 points) (e)Secondly, you should write the code for each class you design in the UML. (15 points) (f)Thirdly, you should write a class named Test which include a main() method, we support there are three clients named Tom(VIPMember), John(NormalMember) and Mike(SuperMember). The class you write can sell a book to the three clients. We support the price of the book is 150.(5 points)
时间: 2024-03-03 12:52:45 浏览: 75
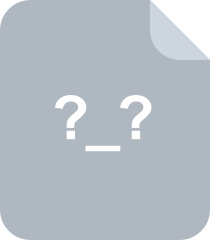
网上图书销售系统uml建模
(a) The Book Seller System should have the following functions:
- Add book to inventory
- Remove book from inventory
- Search for book in inventory
- Sell book to customer
- Calculate the price of a book based on the client's membership level
(b) There are three kinds of clients:
- VIPMember: gets a 10% discount (0.9 * price)
- NormalMember: gets no discount (1 * price)
- SuperMember: gets a 20% discount (0.8 * price)
(c) The strategy pattern will be used to define the different discount strategies for each type of client.
(d) UML class diagram with the strategy pattern:
```
+-------------------------+ +------------------------+
| BookSeller | | MemberStrategy |
+-------------------------+ +------------------------+
| - inventory: List<Book> | | +calculatePrice(price) |
+-------------------------+ +------------------------+
| +addBook(book: Book) | ^
| +removeBook(book: Book) | |
| +searchBook(title: str) | |
| +sellBook(client: Member, | |
| book: Book) | +------------------------+
+-------------------------+ | VIPMemberStrategy |
+------------------------+
| +calculatePrice(price) |
+------------------------+
| NormalMemberStrategy |
+------------------------+
| +calculatePrice(price) |
+------------------------+
| SuperMemberStrategy |
+------------------------+
| +calculatePrice(price) |
+------------------------+
```
(e) Code for each class:
```python
class Book:
def __init__(self, title, author, price):
self.title = title
self.author = author
self.price = price
class Member:
def __init__(self, name, strategy):
self.name = name
self.strategy = strategy
def setStrategy(self, strategy):
self.strategy = strategy
class MemberStrategy:
def calculatePrice(self, price):
pass
class VIPMemberStrategy(MemberStrategy):
def calculatePrice(self, price):
return price * 0.9
class NormalMemberStrategy(MemberStrategy):
def calculatePrice(self, price):
return price
class SuperMemberStrategy(MemberStrategy):
def calculatePrice(self, price):
return price * 0.8
class BookSeller:
def __init__(self):
self.inventory = []
def addBook(self, book):
self.inventory.append(book)
def removeBook(self, book):
self.inventory.remove(book)
def searchBook(self, title):
for book in self.inventory:
if book.title == title:
return book
return None
def sellBook(self, client, book):
price = book.price
price = client.strategy.calculatePrice(price)
print("Selling book {} to {} for price: {}".format(book.title, client.name, price))
# Example usage
book1 = Book("Harry Potter", "J.K. Rowling", 200)
book2 = Book("Lord of the Rings", "J.R.R. Tolkien", 150)
vip = Member("Tom", VIPMemberStrategy())
normal = Member("John", NormalMemberStrategy())
super = Member("Mike", SuperMemberStrategy())
seller = BookSeller()
seller.addBook(book1)
seller.addBook(book2)
seller.sellBook(vip, book1) # Selling book Harry Potter to Tom for price: 180.0
seller.sellBook(normal, book2) # Selling book Lord of the Rings to John for price: 150
seller.sellBook(super, book1) # Selling book Harry Potter to Mike for price: 160.0
```
(f) Test class:
```python
class Test:
def main():
book1 = Book("Harry Potter", "J.K. Rowling", 200)
book2 = Book("Lord of the Rings", "J.R.R. Tolkien", 150)
vip = Member("Tom", VIPMemberStrategy())
normal = Member("John", NormalMemberStrategy())
super = Member("Mike", SuperMemberStrategy())
seller = BookSeller()
seller.addBook(book1)
seller.addBook(book2)
seller.sellBook(vip, book1) # Selling book Harry Potter to Tom for price: 180.0
seller.sellBook(normal, book2) # Selling book Lord of the Rings to John for price: 150
seller.sellBook(super, book1) # Selling book Harry Potter to Mike for price: 160.0
Test.main()
```
阅读全文
相关推荐
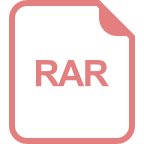
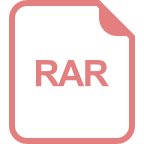
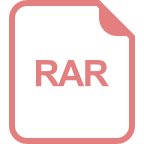
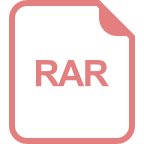
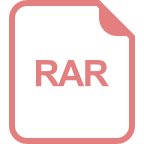
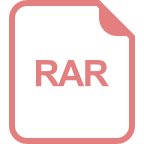