帮我写个安卓蓝牙通信模块
时间: 2023-05-17 12:07:55 浏览: 85
好的,我可以为您提供一些关于安卓蓝牙通信模块的基本信息和代码示例。以下是一个简单的安卓蓝牙通信模块的代码示例:
```
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.os.Handler;
import android.os.Message;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.UUID;
public class BluetoothModule {
private BluetoothAdapter mBluetoothAdapter;
private BluetoothSocket mBluetoothSocket;
private InputStream mInputStream;
private OutputStream mOutputStream;
private Handler mHandler;
public BluetoothModule(Handler handler) {
mHandler = handler;
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
}
public boolean connect(String address) {
BluetoothDevice device = mBluetoothAdapter.getRemoteDevice(address);
UUID uuid = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
try {
mBluetoothSocket = device.createRfcommSocketToServiceRecord(uuid);
mBluetoothSocket.connect();
mInputStream = mBluetoothSocket.getInputStream();
mOutputStream = mBluetoothSocket.getOutputStream();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public void disconnect() {
try {
mBluetoothSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public void write(byte[] bytes) {
try {
mOutputStream.write(bytes);
} catch (IOException e) {
e.printStackTrace();
}
}
public void read() {
byte[] buffer = new byte[1024];
int bytes;
while (true) {
try {
bytes = mInputStream.read(buffer);
String message = new String(buffer, 0, bytes);
Message msg = mHandler.obtainMessage(0, message);
mHandler.sendMessage(msg);
} catch (IOException e) {
e.printStackTrace();
break;
}
}
}
}
```
这个模块包含了连接、断开连接、写入和读取数据的方法。您可以根据需要进行修改和扩展。
阅读全文
相关推荐
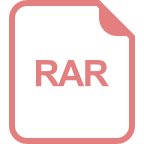
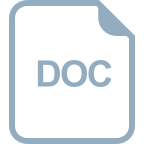

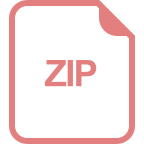
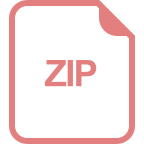
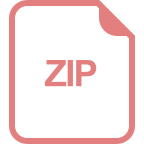
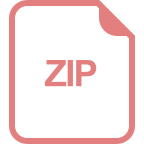
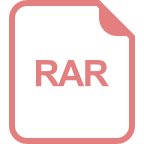
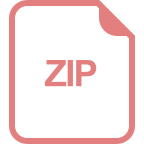
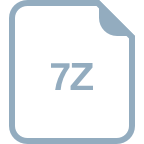
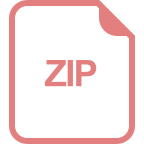
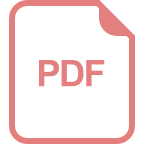
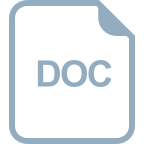


