PyQt5的线程如何获取窗口控件内容,不是发送
时间: 2024-02-26 20:57:22 浏览: 20
在PyQt5中,如果您想在子线程中获取窗口控件的内容而不是发送信号,您可以使用`QMetaObject.invokeMethod()`方法。这个方法可以在主线程中异步调用一个对象的方法,从而避免了直接在子线程中访问窗口控件的问题。
以下是一个例子,演示了如何在子线程中获取窗口控件的内容:
```python
from PyQt5.QtCore import QThread, QMetaObject, pyqtSlot
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout, QLineEdit, QPushButton, QLabel
import sys
class Worker(QThread):
def __init__(self, parent=None):
super().__init__(parent)
def run(self):
# 在这里调用子线程需要执行的任务,例如获取窗口控件内容
content = QMetaObject.invokeMethod(self.parent(), "get_content", Qt.BlockingQueuedConnection)
print("子线程获取到的内容:", content)
class Window(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.resize(400, 300)
self.lineEdit = QLineEdit()
self.pushButton = QPushButton("获取内容")
self.label = QLabel()
layout = QVBoxLayout(self)
layout.addWidget(self.lineEdit)
layout.addWidget(self.pushButton)
layout.addWidget(self.label)
self.pushButton.clicked.connect(self.start_worker)
self.worker = Worker(self)
def start_worker(self):
self.worker.start()
@pyqtSlot()
def get_content(self):
return self.lineEdit.text()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = Window()
window.show()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个`Worker`类,它继承自`QThread`类。在`run`方法中,我们可以执行子线程需要执行的任务,例如使用`QMetaObject.invokeMethod()`方法在主线程中调用`Window`类的`get_content`方法,从而获取窗口控件的内容。
在`Window`类中,我们创建了一个`Worker`实例,并通过构造函数将其父对象设置为`Window`类的实例。我们还定义了一个`get_content`方法,用于获取窗口控件的内容。在`run`方法中,我们使用`QMetaObject.invokeMethod()`方法异步调用`get_content`方法,并将其返回值存储在`content`变量中。
这种方法可以避免在子线程中直接访问窗口控件的问题,但需要注意的是,由于使用了异步调用,`get_content`方法必须是线程安全的,否则可能会导致竞态条件和数据不一致的问题。
相关推荐
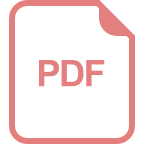
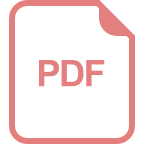
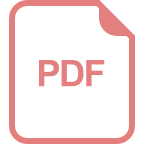














