用C++币安订阅aggtrade例子
时间: 2024-04-06 07:32:07 浏览: 11
以下是用C++连接币安API订阅aggtrade的例子,可以参考:
```cpp
#include <iostream>
#include <sstream>
#include <vector>
#include <string>
#include <thread>
#include <chrono>
#include <openssl/hmac.h>
#include <curl/curl.h>
#include <rapidjson/document.h>
using namespace rapidjson;
const std::string api_key = "YOUR_API_KEY";
const std::string secret_key = "YOUR_SECRET_KEY";
const std::string base_url = "https://api.binance.com";
const std::string stream_url = "wss://stream.binance.com:9443/ws";
std::string get_signature(const std::string& message, const std::string& secret) {
unsigned char hmac[32];
HMAC(EVP_sha256(), secret.c_str(), secret.length(), (const unsigned char*)message.c_str(), message.length(), hmac, NULL);
std::stringstream ss;
for (int i = 0; i < 32; ++i) {
ss << std::hex << std::setw(2) << std::setfill('0') << (int)hmac[i];
}
return ss.str();
}
void subscribe_aggtrade(const std::string& symbol) {
std::string path = "/api/v3/userDataStream";
std::string url = base_url + path;
CURL* curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
struct curl_slist* headers = NULL;
std::string timestamp = std::to_string(std::chrono::duration_cast<std::chrono::milliseconds>(std::chrono::system_clock::now().time_since_epoch()).count());
std::string message = "timestamp=" + timestamp;
std::string signature = get_signature(message, secret_key);
headers = curl_slist_append(headers, ("X-MBX-APIKEY: " + api_key).c_str());
headers = curl_slist_append(headers, ("Content-Type: application/x-www-form-urlencoded").c_str());
std::string post_fields = "timestamp=" + timestamp + "&signature=" + signature;
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, post_fields.c_str());
std::string response;
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, [](char* ptr, size_t size, size_t count, void* userdata) {
std::string* response = (std::string*)userdata;
response->append(ptr, size * count);
return size * count;
});
CURLcode res = curl_easy_perform(curl);
if (res == CURLE_OK) {
Document d;
d.Parse(response.c_str());
std::string listen_key = d["listenKey"].GetString();
path = "/ws/" + listen_key;
url = stream_url + path;
headers = NULL;
headers = curl_slist_append(headers, ("X-MBX-APIKEY: " + api_key).c_str());
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, [](char* ptr, size_t size, size_t count, void* userdata) {
std::string* response = (std::string*)userdata;
response->append(ptr, size * count);
size_t npos = response->find("\r\n");
while (npos != std::string::npos) {
std::string message = response->substr(0, npos);
response->erase(0, npos + 2);
std::cout << message << std::endl;
npos = response->find("\r\n");
}
return size * count;
});
curl_easy_perform(curl);
}
curl_easy_cleanup(curl);
}
}
int main() {
subscribe_aggtrade("btcusdt");
return 0;
}
```
在这个例子中,我们首先通过调用 `/api/v3/userDataStream` 接口获取 listenKey,然后使用 WebSocket 连接订阅指定 symbol 的 aggtrade 数据。订阅的数据会通过 WebSocket 推送,我们在 `CURLOPT_WRITEFUNCTION` 中处理推送过来的数据。
需要注意的是,这个例子中使用的是币安的永久用户数据流,需要通过 `/api/v3/userDataStream` 接口获取 listenKey。如果不使用永久用户数据流,可以使用 `stream.binance.com` 替换为 `stream.binancefuture.com` 或者 `fstream.binance.com`,并在连接 WebSocket 时在 URL 中加上 `@aggTrade`,例如:
```
wss://fstream.binance.com/ws/btcusdt@aggTrade
```
同时,这个例子中使用了 OpenSSL 和 libcurl 库,需要在编译时链接这些库,例如:
```
g++ -std=c++11 -o test test.cpp -lcrypto -lcurl
```
相关推荐
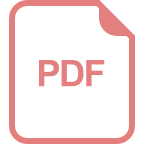
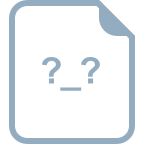














