python飞机大战中飞机被击中发生爆炸特效的代码
时间: 2023-11-12 16:44:15 浏览: 234
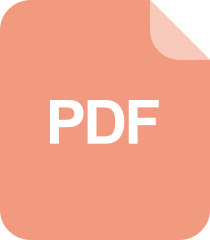
使用python实现飞机大战游戏

在 Python 飞机大战游戏中,可以使用 Pygame 库来实现飞机被击中发生爆炸特效的效果。以下是一个简单的实现代码示例:
首先,需要导入 Pygame 库并初始化:
```python
import pygame
pygame.init()
```
然后,定义一个 `Explosion` 类来表示爆炸特效。该类继承自 Pygame 的 `Sprite` 类,因此可以方便地与游戏场景中的其他元素进行交互。在该类的 `__init__` 方法中,需要加载爆炸动画的所有帧,并将它们存储在一个列表中。在 `update` 方法中,每次更新爆炸动画的帧索引,并在最后一帧后将该特效从场景中移除。
```python
class Explosion(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.images = []
for i in range(9):
img = pygame.image.load(f"explosion{i}.png").convert_alpha()
img = pygame.transform.scale(img, (64, 64))
self.images.append(img)
self.image_index = 0
self.image = self.images[self.image_index]
self.rect = self.image.get_rect()
self.rect.center = (x, y)
def update(self):
self.image_index += 1
if self.image_index >= len(self.images):
self.kill()
else:
self.image = self.images[self.image_index]
```
当飞机被击中时,可以创建一个 `Explosion` 对象,并将其添加到游戏场景中的爆炸特效组中。在每次游戏循环中,需要更新并绘制该组中的所有特效。
```python
explosion_group = pygame.sprite.Group()
# 在飞机被击中处添加以下代码
explosion = Explosion(plane.rect.centerx, plane.rect.centery)
explosion_group.add(explosion)
# 在游戏循环中添加以下代码
explosion_group.update()
explosion_group.draw(screen)
```
最后,当游戏结束时,需要清除爆炸特效组中的所有特效,以便在下一次游戏中重新使用。
```python
explosion_group.empty()
```
阅读全文
相关推荐
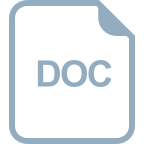
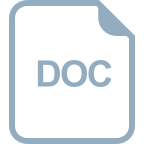
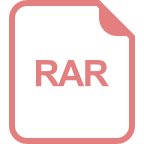
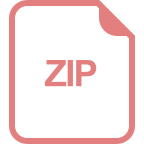
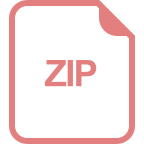
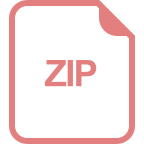
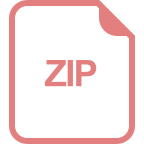
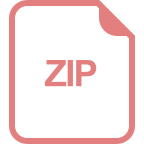
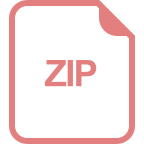
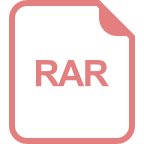
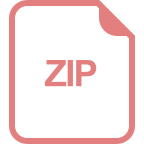
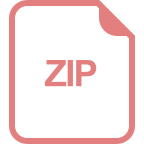
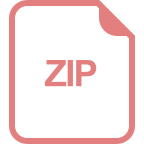
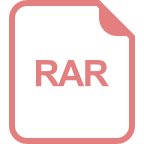
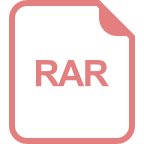
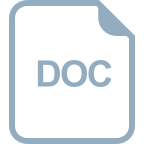
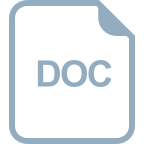
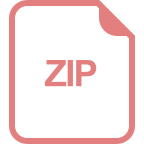
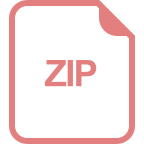