nn.sequential()构建cnn模型中加入注意力机制用于图片分类
时间: 2023-07-04 12:15:38 浏览: 56
要在CNN模型中加入注意力机制,可以使用PyTorch中提供的torchvision包中的预训练模型,并对其进行微调,或者自己构建模型并加入注意力机制。
如果你想自己构建模型并加入注意力机制,可以使用nn.Sequential()构建模型,并在其中加入注意力机制。在PyTorch中,可以使用nn.MultiheadAttention()或者nn.SelfAttention()添加注意力机制。具体实现方法如下:
```python
import torch
import torch.nn as nn
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Sequential(
nn.Conv2d(3, 64, 3, 1, 1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, 3, 1, 1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(2, 2)
)
self.conv2 = nn.Sequential(
nn.Conv2d(64, 128, 3, 1, 1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, 3, 1, 1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.MaxPool2d(2, 2)
)
self.conv3 = nn.Sequential(
nn.Conv2d(128, 256, 3, 1, 1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, 3, 1, 1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, 3, 1, 1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.MaxPool2d(2, 2)
)
self.attention = nn.MultiheadAttention(embed_dim=256, num_heads=4)
self.fc = nn.Sequential(
nn.Linear(256*4*4, 512),
nn.ReLU(inplace=True),
nn.Linear(512, 10)
)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = self.conv3(x)
# 添加注意力机制
x = x.permute(2, 3, 0, 1) # 调整维度顺序
x, _ = self.attention(x, x, x)
x = x.permute(2, 3, 0, 1) # 调整维度顺序
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
在上述代码中,我们使用了nn.MultiheadAttention()添加了注意力机制。首先,我们需要将卷积层输出的特征图的维度顺序调整为(batch, channel, height, width),然后输入到nn.MultiheadAttention()中进行处理,并将输出的特征图维度顺序再次调整为(height, width, batch, channel),最后再接上全连接层进行分类。
请注意,注意力机制的输入和输出都是二维张量,因此需要将卷积层输出的特征图转换为二维张量,即将维度为(batch, channel, height, width)的特征图展开成(batch, channel*height*width)的二维张量,这里使用了x.view(x.size(0), -1)来实现。
相关推荐
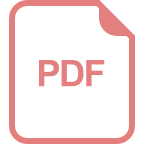
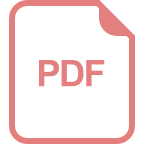
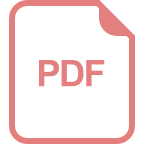














