library ieee; use ieee.std_logic_1164.all; use ieee.std_logic_unsigned.all; entity divide_25 is port(clk_in : in std_logic; clk_out : out std_logic); end; architecture fenpin of divide_25 is signal cnt :integer range 0 to 24; signal clk_tmp : std_logic; begin process(clk_in) begin if(clk_in'event and clk_in='1')then if cnt=24 then cnt<=0; clk_tmp<=not clk_tmp; else cnt<=cnt+1; end if; end if; end process; clk_out<=clk_tmp; end;翻译
时间: 2024-03-30 11:38:55 浏览: 151
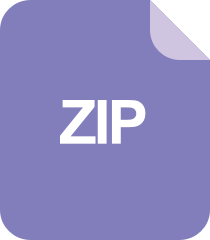
std_logic_arith.zip_logic_std_logic_arith_vhdl
这段代码是一个基于时钟的分频器,输入一个时钟信号clk_in,输出一个分频后的时钟信号clk_out。其中,分频比为25,即输出时钟频率为输入时钟频率的1/25。代码中使用了IEEE标准库中的std_logic_1164和std_logic_unsigned库。在architecture部分,定义了一个计数器cnt,范围为0到24,用来计数分频比。同时,定义了一个辅助时钟信号clk_tmp,用来生成分频后的时钟信号。在process过程中,当输入时钟信号上升沿时,若计数器cnt已经达到24,则将计数器复位为0,并将辅助时钟信号clk_tmp取反;否则,将计数器加1。最后,将辅助时钟信号clk_tmp赋值给输出时钟信号clk_out。
阅读全文
相关推荐
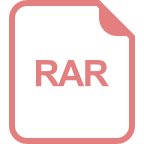
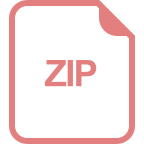


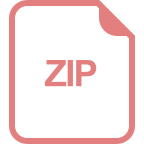
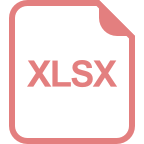
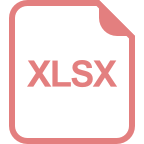
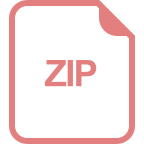
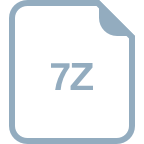
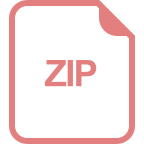
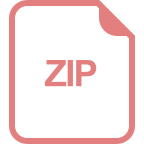
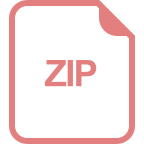
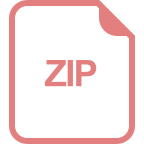