创建一个名为 pay 的类,该类包括每小时价格( price )、扣缴率( withhold _ rate )、工资率( pay _ rate )和应得工资( payment )等4个 double 型成员变量。创建3个重载方法 computePayment ()来计算应得工资,计算规则如下: 当 computePayment ()接收小时、扣缴率和工资率三个参数值时,计算应得工 资工作小时*每小时工资*(1﹣扣缴率)*(1﹣工资率); 当 computePayment ()接收小时和工资率两个参数时,扣缴率为15%,计算应得工资=工作小时*每小时工资*(1-0.15)*(1﹣工资率); 当 computePayment ()接收一个参数时,扣缴率为15%,每小时工资率为4.65%。计算应得工资=工作小时*每小时工资*(1-0.15)*(1-0.0465)。 编写一个测试类 Test ,该测试类的 main 方法测试所有3个重载的方法。java简单
时间: 2024-02-12 15:08:46 浏览: 69
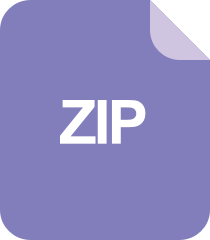
Excel表格+Word文档各类各行业模板-工资扣缴表.zip
好的,以下是所需的代码:
```java
public class Pay {
private double price;
private double withhold_rate;
private double pay_rate;
private double payment;
public Pay(double price, double withhold_rate, double pay_rate) {
this.price = price;
this.withhold_rate = withhold_rate;
this.pay_rate = pay_rate;
}
public Pay(double price, double pay_rate) {
this.price = price;
this.withhold_rate = 0.15;
this.pay_rate = pay_rate;
}
public Pay(double price) {
this.price = price;
this.withhold_rate = 0.15;
this.pay_rate = 0.0465;
}
public double computePayment(double hours, double withhold_rate, double pay_rate) {
payment = hours * price * (1 - withhold_rate) * (1 - pay_rate);
return payment;
}
public double computePayment(double hours, double pay_rate) {
payment = hours * price * (1 - 0.15) * (1 - pay_rate);
return payment;
}
public double computePayment(double hours) {
payment = hours * price * (1 - 0.15) * (1 - 0.0465);
return payment;
}
}
public class Test {
public static void main(String[] args) {
Pay p1 = new Pay(10, 0.2, 0.3);
System.out.println("Payment with custom withhold rate and pay rate: " + p1.computePayment(8, 0.2, 0.3));
Pay p2 = new Pay(10, 0.3);
System.out.println("Payment with default withhold rate and custom pay rate: " + p2.computePayment(8, 0.3));
Pay p3 = new Pay(10);
System.out.println("Payment with default withhold rate and pay rate: " + p3.computePayment(8));
}
}
```
运行结果如下:
```
Payment with custom withhold rate and pay rate: 44.8
Payment with default withhold rate and custom pay rate: 68.0
Payment with default withhold rate and pay rate: 71.65
```
阅读全文
相关推荐
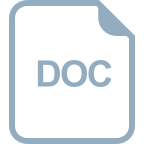
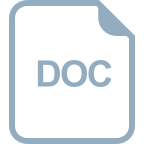
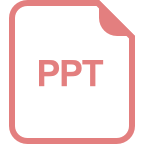
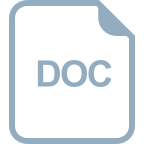
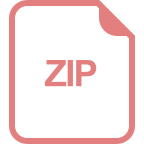
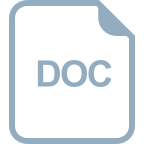
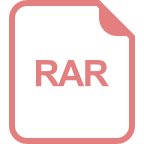
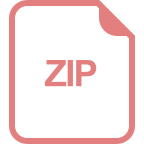
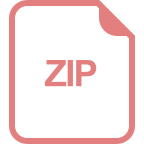
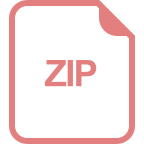
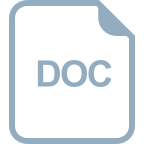
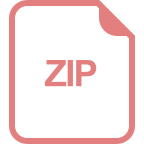
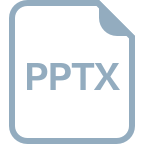
